1# LibSQLStudio
2
3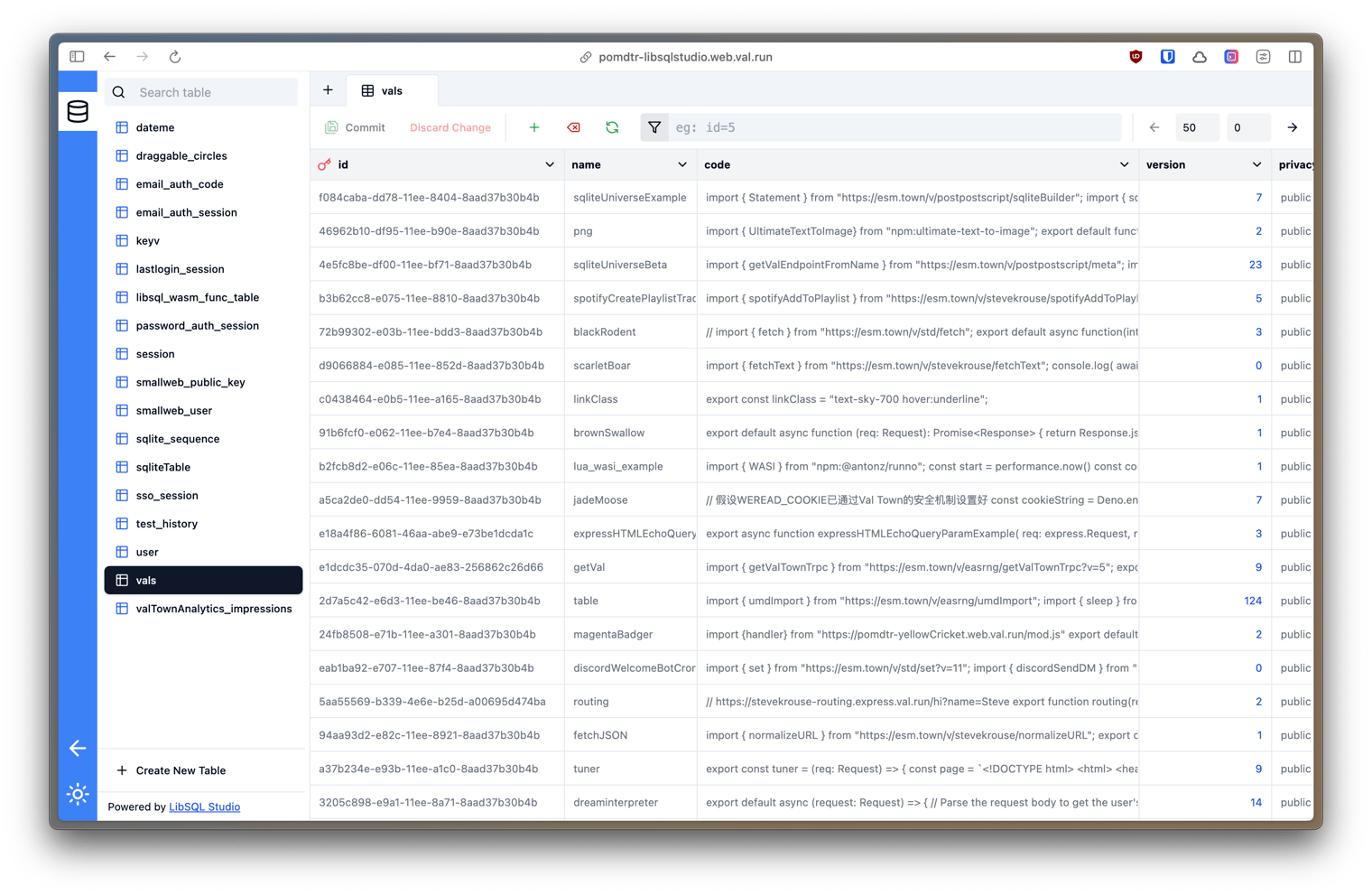
4
5To authenticate, use an [API Token](https://www.val.town/settings/api).
3View and interact with your Val Town SQLite data. It's based off Steve's excellent [SQLite Admin](https://www.val.town/v/stevekrouse/sqlite_admin?v=46) val, adding the ability to run SQLite queries directly in the interface. This new version has a revised UI and that's heavily inspired by [LibSQL Studio](https://github.com/invisal/libsql-studio) by [invisal](https://github.com/invisal). This is now more an SPA, with tables, queries and results showing up on the same page.
4
5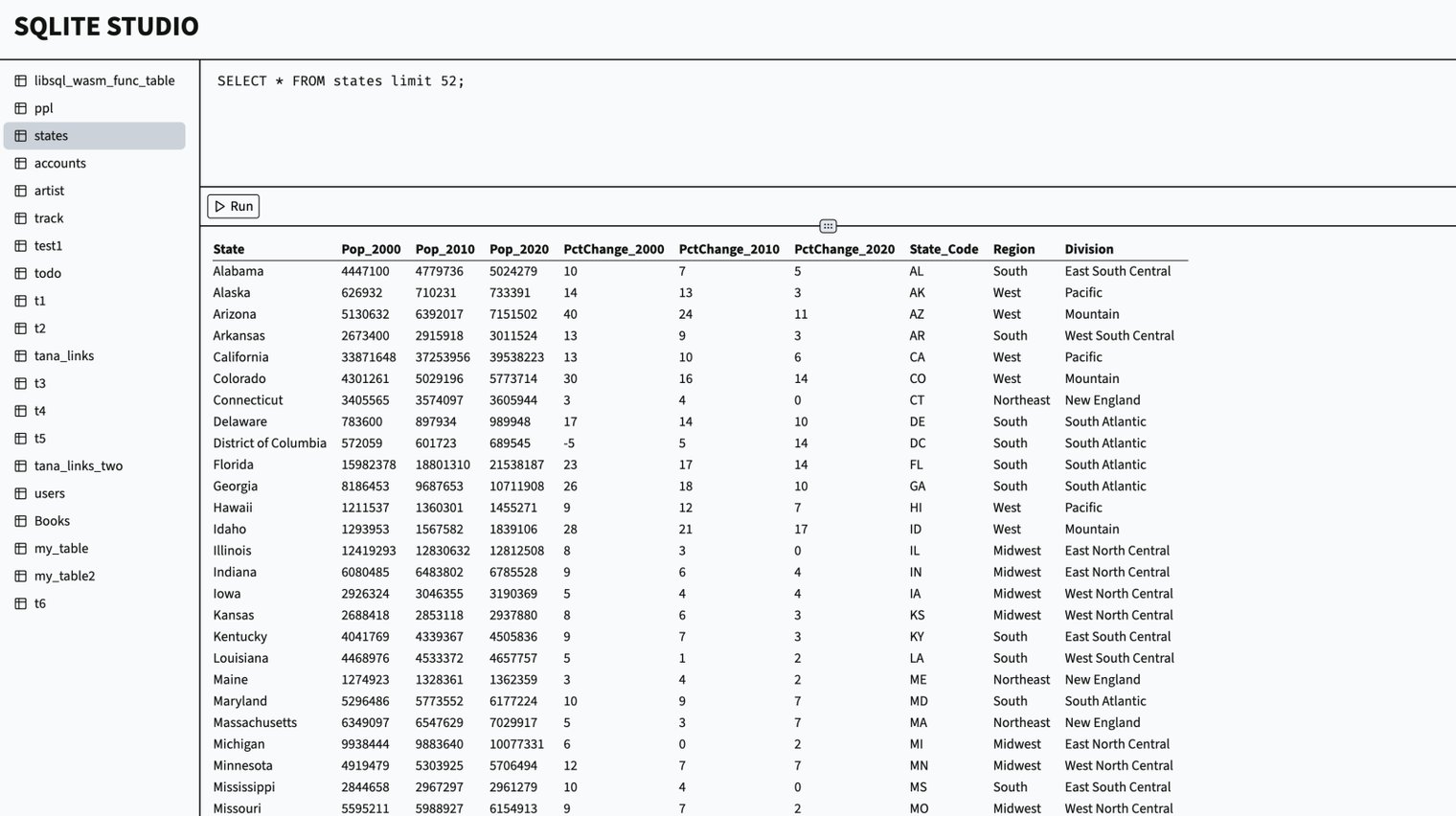
6
7## Install
5
6async function run() {
7 const imageUrl =
8 "https://prodimage.images-bn.com/lf?set=key%5Bresolve.pixelRatio%5D,value%5B1%5D&set=key%5Bresolve.width%5D,value%5B600%5D&set=key%5Bresolve.height%5D,value%5B10000%5D&set=key%5Bresolve.imageFit%5D,value%5Bcontainerwidth%5D&set=key%5Bresolve.allowImageUpscaling%5D,value%5B0%5D&set=key%5Bresolve.format%5D,value%5Bwebp%5D&source=url%5Bhttps://prodimage.images-bn.com/pimages/9780063345164_p0_v3_s600x595.jpg%5D&scale=options%5Blimit%5D,size%5B600x10000%5D&sink=format%5Bwebp%5D";
9 console.log("ran");
10
11 try {
12 await axios.get(imageUrl, { responseType: "arraybuffer" });
13 console.log("ran axios");
14 } catch (error) {
22 const scriptCount = (html.match(/<script/g) || []).length;
23 const styleCount = (html.match(/<style/g) || []).length + (html.match(/<link[^>]*stylesheet/g) || []).length;
24 const imageCount = (html.match(/<img/g) || []).length;
25
26 const jsSize = html.match(/<script[^>]*>([\s\S]*?)<\/script>/g)?.reduce((total, script) => total + script.length, 0) || 0;
37 scriptCount,
38 styleCount,
39 imageCount,
40 jsSize,
41 cssSize,
104 Script Count: ${r.scriptCount}
105 Style Count: ${r.styleCount}
106 Image Count: ${r.imageCount}
107 Estimated JS Size: ${r.jsSize} bytes
108 Estimated CSS Size: ${r.cssSize} bytes
1import { fetchJSON } from "https://esm.town/v/stevekrouse/fetchJSON";
2
3export const nasaImageDetails = async () => {
4 const nasaAPOD = await fetchJSON("https://api.nasa.gov/planetary/apod?api_key=DEMO_KEY");
5 let nasaImageHtml = nasaAPOD.hdurl
6 ? `<img width="100%" src="${nasaAPOD.hdurl}"/>`
7 : nasaAPOD.media_type === "video"
8 ? `<iframe width="100%" height="100%" src="${nasaAPOD.url}" title="YouTube video player" frameborder="0" allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture; web-share" allowfullscreen></iframe>`
9 : `<p>Unknown Type</p><br/><code><pre>${JSON.stringify(nasaAPOD, null, 2)}</pre></code>`;
10 nasaImageHtml += `<p>${nasaAPOD.explanation}</p>`;
11 nasaImageHtml += "<p>"
12 + (nasaAPOD.media_type === "video"
13 ? `<small>Video Url: ${nasaAPOD.url}</small>`
14 : `<p><small>Image Url: ${nasaAPOD.hdurl}</small>`)
15 + "</p>";
16 return { html: nasaImageHtml, nasaAPOD };
17};
3Move circles around. State is synced with the server. Open a window in another tab and watch the circles update as you move them .
4
5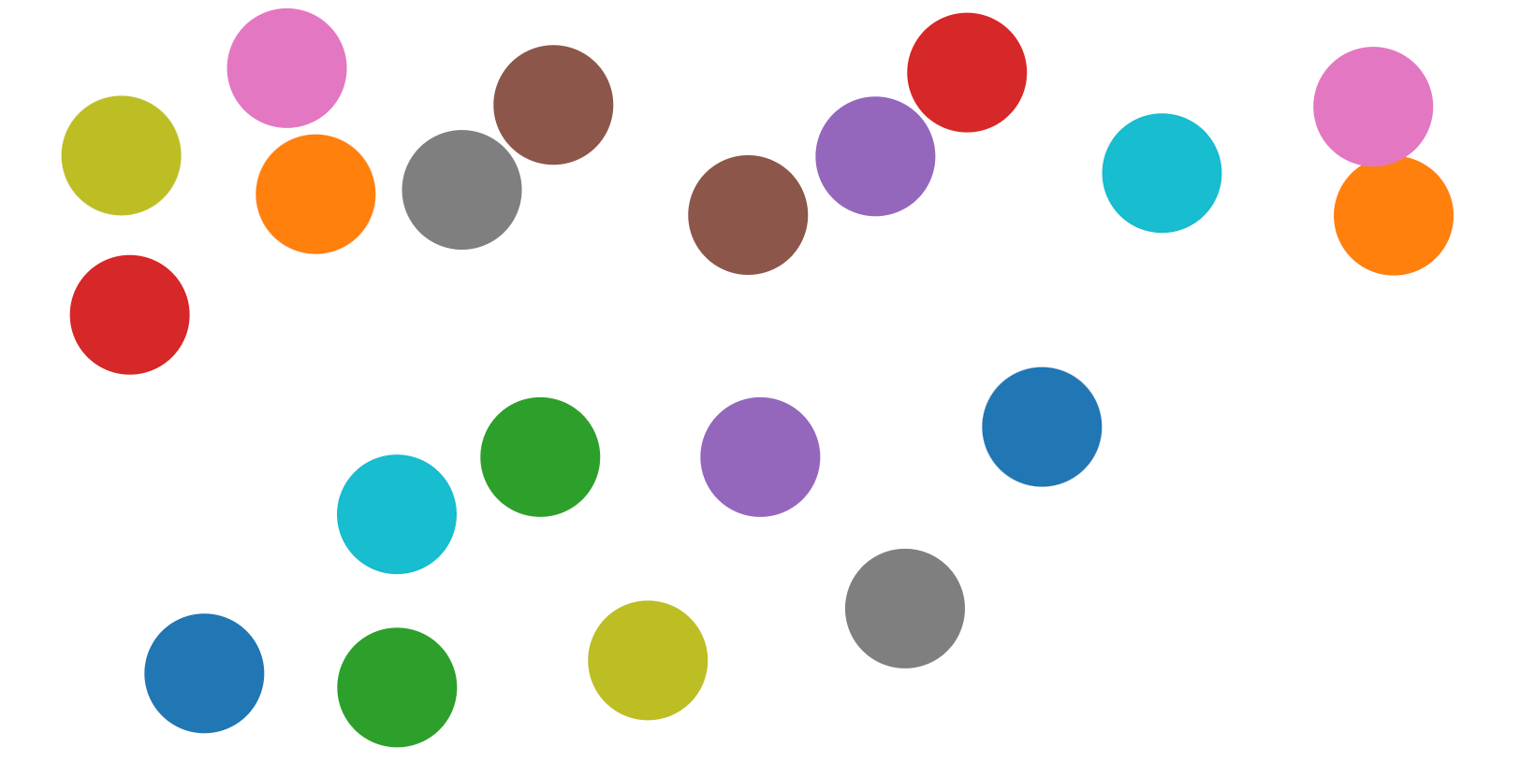
6
5 const description = "social meta test";
6 const title = "JGB Test";
7 const image = "https://jesusgollonet.com/images/social/back-to-blocked.png";
8 return new Response(
9 renderToString(
14 <meta property="og:title" content={title} />
15 <meta property="og:description" content={description} />
16 <meta property="og:image" content={image} />
17 {/* twitter card*/}
18 <meta name="twitter:card" content="summary_large_image" />
19 <meta name="twitter:site" content="@jesusgollonet" />
20 <meta name="twitter:creator" content="@jesusgollonet" />
21 <meta name="twitter:title" content={title} />
22 <meta name="twitter:description" content={description} />
23 <meta name="twitter:image" content={image} />
24 <link rel="icon" href="/favicon.ico" />
25
1# Blob Storage - [Docs ↗](https://docs.val.town/std/blob)
2
3Val Town comes with blob storage built-in. It allows for storing any data: text, JSON, images. You can access it via [`std/blob`](https://www.val.town/v/std/blob).
4
5Blob storage is scoped globally to your account. If you set a blob in one val, you can retrieve it by the same key in another val. It's backed by Cloudflare R2.
5/**
6 * Provides functions for interacting with your account's blob storage.
7 * Blobs can store any data type (text, JSON, images, etc.) and allow
8 * retrieval across different vals using the same key.
9 * ([Docs ↗](https://docs.val.town/std/blob))
9const app = new Hono();
10
11const DOWNLOAD_URL = "https://yawnxyz-serve.web.val.run"; // https://yawnxyz-serve.web.val.run/myImage.jpg
12// Define an array of JSON objects
13let blobbyList = await blobby.list();
428 <form class="input-btn-group | flex flex-row gap-0" @submit.prevent="uploadFromUrl(item.key)">
429 <button type="submit" class="flex items-center rounded-l-md px-4 py-1 text-sm cursor-pointer bg-gray-100 hover:bg-gray-200">Upload from Url</button>
430 <input x-text="blobs[item.key]?.uploadUrl" type="text" class="rounded-l-xs rounded-r-md border-1 border-gray-200 px-4 py-1 text-sm text-gray-800 bg-gray-100" @input="updateBlobUrl(item.key, $event.target.value)" placeholder="https://image/mp3/etc">
431 </form>
432 </div>
461 </template>
462
463 <template x-if="blobs[item.key]?.type?.includes('image')">
464 <img :src="blobs[item.key]?.url" controls></audio>
465 </template>
643 <form class="input-btn-group flex flex-row gap-0" @submit.prevent="uploadFromUrl">
644 <button type="submit" class="flex items-center rounded-l-md px-4 py-1 text-sm cursor-pointer bg-gray-100 hover:bg-gray-200">Upload from Url</button>
645 <input x-model="uploadUrl" type="text" class="rounded-l-xs rounded-r-md border-1 border-gray-200 px-4 py-1 text-sm text-gray-800 bg-gray-100" placeholder="https://image/mp3/etc">
646 </form>
647 </div>
676 </template>
677
678 <template x-if="blobType && blobType.includes('image')">
679 <img :src="blobUrl" alt="Blob content">
680 </template>