2import React, { useEffect, useRef, useState } from "https://esm.sh/react@18.2.0";
34export function RandomPositionImage({
5imageUrl = "https://maxm-imggenurl.web.val.run/random-colorful-abstract-shape",
6existingPositions = [],
7}) {
8const [position, setPosition] = useState({ top: 0, left: 0 });
9const [size, setSize] = useState({ width: 250, height: 250 });
10const [imageLoaded, setImageLoaded] = useState(false);
11const [isDragging, setIsDragging] = useState(false);
12const [dragOffset, setDragOffset] = useState({ x: 0, y: 0 });
113width: `${size.width}`,
114height: `auto`,
115opacity: imageLoaded ? 1 : 0,
116transition: isDragging ? "none" : "opacity 0.3s ease-in-out",
117cursor: isDragging ? "grabbing" : "grab",
121<img
122ref={imgRef}
123src={imageUrl}
124alt="Random Generated Image"
125onLoad={() => setImageLoaded(true)}
126style={{
127width: "100%",
3import { DATABASE_TABLENAME } from "./constants";
45// export async function getImages(type: string, count: number): Promise<string[]> {
6// // Query to select random rows of the specified type
7// const result = await sqlite.execute(
16// );
1718// let images: string[] = [];
19// for (const row of result.rows) {
20// // console.log("row", row);
21// // const blobUrl = await blob.get(row["path"] as string);
22// images.push(row["path"] as string);
23// }
2425// return images;
26// }
2728export async function getImages(typeCountMap: Record<string, number>): Promise<Array<string>> {
29const params: (string | number)[] = [];
304142console.log(
43await getImages({
44"image": 10,
45"gif": 5,
46}),
7const [uploadStatus, setUploadStatus] = useState<string>("Drag and drop files here");
8const [fileUrl, setFileUrl] = useState<string | null>(null);
9const [imageType, setImageType] = useState<string>("image");
10const urlInputRef = useRef<HTMLInputElement>(null);
112930// Validate file type
31if (!["image/jpeg", "image/gif", "image/png"].includes(file.type)) {
32setUploadStatus("Only JPG, GIF, and PNG files are allowed");
33return;
36const formData = new FormData();
37formData.append("file", file);
38formData.append("type", imageType);
3940fetch("/upload", {
52});
53}
54}, [imageType]);
5556const handleDragOver = useCallback((event: React.DragEvent<HTMLDivElement>) => {
77<p>{uploadStatus}</p>
78<select
79value={imageType}
80onChange={(e) => setImageType(e.target.value)}
81style={{
82width: "100%",
85}}
86>
87<option value="image">Image</option>
88<option value="gif">GIF</option>
89<option value="stock_image">Stock Image</option>
90</select>
91<div
97}}
98>
99Drag and drop an image here
100</div>
101{fileUrl && (
146}}
147>
148View Image
149</a>
150</div>
167const formData = await request.formData();
168const file = formData.get("file") as File;
169const imageType = formData.get("type") as string;
170171if (!file) {
204await sqlite.execute(
205`INSERT INTO ${DATABASE_TABLENAME} (type, path) VALUES (?, ?)`,
206[imageType, publicUrl],
207);
208211url: publicUrl,
212filename: filename,
213type: imageType,
214}),
215{
223<html>
224<head>
225<title>Image Upload</title>
226<style>${css}</style>
227</head>
valentines_day_card_generatorindex5 matches
1import { getImages } from "./get_images";
23export default async function server(request: Request): Promise<Response> {
4const url = new URL(request.url);
5if (request.method === "GET" && url.pathname === "/image") {
6const params = url.searchParams;
714console.log("typecountmpap", typeCountMap);
1516const imageUrls = await getImages(typeCountMap);
1718return new Response(
19JSON.stringify({
20urls: imageUrls,
21}),
22{
32<html>
33<head>
34<title>Random Image Placement</title>
35<meta name="viewport" content="width=device-width, initial-scale=1">
36<style>
3import React, { useEffect, useRef, useState } from "https://esm.sh/react@18.2.0";
45// import { getImages } from "./get-images";
67// const images = await getImages("image", 12);
8// export const IMAGE_URLS = images;
910// mock data
11const IMAGE_URLS = [
12"https://charmaine-blob_admin.web.val.run/api/public/public%2F1738957396306_pngtree-valentine-day-gift-box-open-love-heart-and-rose-potale-greeting-png-image_6564851.png",
13"https://charmaine-blob_admin.web.val.run/api/public/public%2F1738959510139_2020-heart-pounding-animation.gif",
14"https://charmaine-blob_admin.web.val.run/api/public/public%2F1738959545863_sugary-heart-valentine-png-5692911.png",
15"https://charmaine-blob_admin.web.val.run/api/public/public%2F1738957543311_Red_Happy_Valentine's_Day_PNG_Clip-Art_Image.png",
16];
1781}
8283function DraggableImage({ imageUrl, initialPosition, index, onPositionChange, isCreating, delay = 0 }) {
84const [position, setPosition] = useState(initialPosition);
85const [isDragging, setIsDragging] = useState(false);
149>
150<img
151src={imageUrl}
152alt="Valentine"
153style={{
165const [message, setMessage] = useState("");
166const [isCreating, setIsCreating] = useState(false);
167const [imagePositions, setImagePositions] = useState([]);
168const [showEnvelope, setShowEnvelope] = useState(false);
169const [contentVisible, setContentVisible] = useState(false);
177if (urlMessage) setMessage(decodeURIComponent(urlMessage));
178if (urlPositions) {
179setImagePositions(JSON.parse(decodeURIComponent(urlPositions)));
180} else {
181initializeRandomPositions();
196scale: 0.8 + Math.random() * 0.4,
197}));
198setImagePositions(positions);
199};
200201const handlePositionChange = (index, newPosition) => {
202const newPositions = [...imagePositions];
203newPositions[index] = newPosition;
204setImagePositions(newPositions);
205};
206208const url = new URL(window.location.href);
209url.searchParams.set("message", message);
210url.searchParams.set("positions", encodeURIComponent(JSON.stringify(imagePositions)));
211navigator.clipboard.writeText(url.toString());
212alert("URL copied to clipboard!");
278/>
279<div style={{ fontSize: "14px", color: "#666" }}>
280Drag the images to arrange them
281</div>
282<button
347)}
348349{imagePositions.map((position, index) => (
350<DraggableImage
351key={index}
352index={index}
353imageUrl={IMAGE_URLS[index % IMAGE_URLS.length]}
354initialPosition={position}
355onPositionChange={handlePositionChange}
45// export const manager = {
6// getImages(type: string, count: number): Promise<string[]> {
7// return getImages(type, count);
8// },
9// };
1011export async function getImages(type: string, count: number): Promise<string[]> {
12// Query to select random rows of the specified type
13const result = await sqlite.execute(
22);
2324let images: string[] = [];
25console.log(result.rows);
26for (const row of result.rows) {
27const blobPath = await blob.get(row["path"] as string);
28images.push(`https://shouser-blob_admin.web.val.run/api/public/${blobPath.url}`);
29}
3031return images;
32// console.log("images", await getImages("image", 1));
33// Extract paths from the result rows
34// return result.rows.map(asyncrow => await blob.get(row.path as string));
35}
3637console.log(await getImages("image", 10));
codemirrorTsREADME.md1 match
234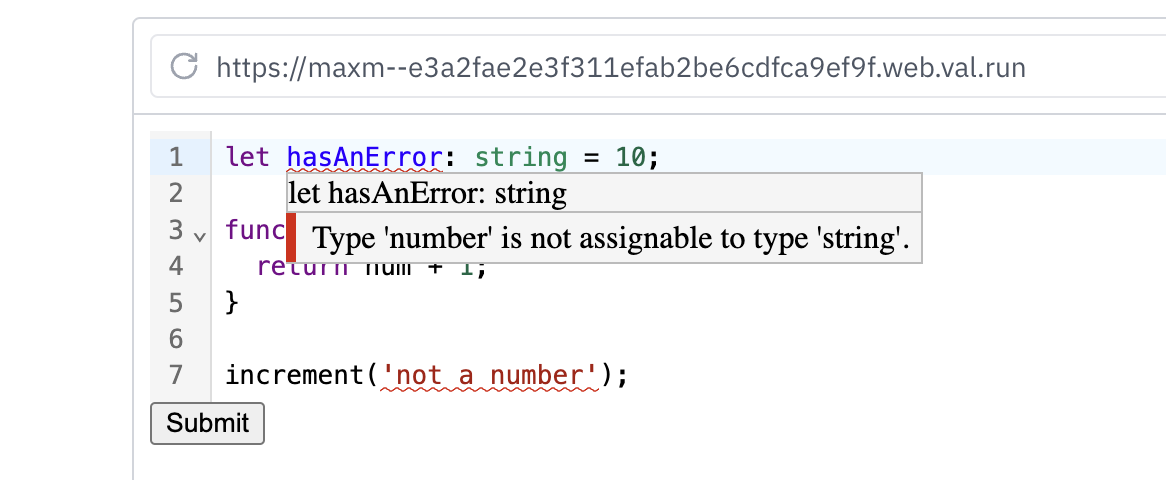
56
45// export const manager = {
6// getImages(type: string, count: number): Promise<string[]> {
7// return getImages(type, count);
8// },
9// };
1011export async function getImages(type: string, count: number): Promise<string[]> {
12// Query to select random rows of the specified type
13const result = await sqlite.execute(
22);
2324// console.log("images", await getImages("image", 1));
25// Extract paths from the result rows
26return result.rows.map(row => await blob.get(row.path));
7const [uploadStatus, setUploadStatus] = useState<string>("Drag and drop files here");
8const [fileUrl, setFileUrl] = useState<string | null>(null);
9const [imageType, setImageType] = useState<string>("image");
10const urlInputRef = useRef<HTMLInputElement>(null);
112930// Validate file type
31if (!["image/jpeg", "image/gif", "image/png"].includes(file.type)) {
32setUploadStatus("Only JPG, GIF, and PNG files are allowed");
33return;
36const formData = new FormData();
37formData.append("file", file);
38formData.append("type", imageType);
3940fetch("/upload", {
52});
53}
54}, [imageType]);
5556const handleDragOver = useCallback((event: React.DragEvent<HTMLDivElement>) => {
77<p>{uploadStatus}</p>
78<select
79value={imageType}
80onChange={(e) => setImageType(e.target.value)}
81style={{
82width: "100%",
85}}
86>
87<option value="image">Image</option>
88<option value="gif">GIF</option>
89<option value="stock_image">Stock Image</option>
90</select>
91<div
97}}
98>
99Drag and drop an image here
100</div>
101{fileUrl && (
146}}
147>
148View Image
149</a>
150</div>
167const formData = await request.formData();
168const file = formData.get("file") as File;
169const imageType = formData.get("type") as string;
170171if (!file) {
204await sqlite.execute(
205`INSERT INTO ${DATABASE_TABLENAME} (type, path) VALUES (?, ?)`,
206[imageType, filename],
207);
208211url: publicUrl,
212filename: filename,
213type: imageType,
214}),
215{
223<html>
224<head>
225<title>Image Upload</title>
226<style>${css}</style>
227</head>
3hi sophieee
45ok so i only edited `frontend_card`, you can see where im directly grabbing a public url i have in my blob storage aka: https://charmaine-blob_admin.web.val.run/api/public/public%2F1738957543311_Red_Happy_Valentine's_Day_PNG_Clip-Art_Image.png
67i would love to be able to make get requests to a url like that instead of hard coding them :p