stevensDemo_1generateFunFacts.ts6 matches
2import { nanoid } from "https://esm.sh/nanoid@5.0.5";
3import { sqlite } from "https://esm.town/v/stevekrouse/sqlite";
4import { Configuration, OpenAIApi } from "npm:openai";
56const TABLE_NAME = `memories`;
77async function generateFunFacts(previousFacts) {
78try {
79const apiKey = Deno.env.get("OPENAI_API_KEY");
80if (!apiKey) {
81console.error("OpenAI API key is not configured.");
82return null;
83}
8485const configuration = new Configuration({ apiKey });
86const openai = new OpenAIApi(configuration);
8788const previousFactsText = previousFacts
blob_adminmain.tsx6 matches
1516// Public route without authentication
17app.get("/api/public/:id", async (c) => {
18const key = `__public/${c.req.param("id")}`;
19const { blob } = await import("https://esm.town/v/std/blob");
133};
134135app.get("/api/blobs", checkAuth, async (c) => {
136const prefix = c.req.query("prefix") || "";
137const limit = parseInt(c.req.query("limit") || "20", 10);
142});
143144app.get("/api/blob", checkAuth, async (c) => {
145const key = c.req.query("key");
146if (!key) return c.text("Missing key parameter", 400);
150});
151152app.put("/api/blob", checkAuth, async (c) => {
153const key = c.req.query("key");
154if (!key) return c.text("Missing key parameter", 400);
159});
160161app.delete("/api/blob", checkAuth, async (c) => {
162const key = c.req.query("key");
163if (!key) return c.text("Missing key parameter", 400);
167});
168169app.post("/api/blob", checkAuth, async (c) => {
170const { file, key } = await c.req.parseBody();
171if (!file || !key) return c.text("Missing file or key", 400);
blob_adminapp.tsx19 matches
70const menuRef = useRef(null);
71const isPublic = blob.key.startsWith("__public/");
72const publicUrl = isPublic ? `${window.location.origin}/api/public/${encodeURIComponent(blob.key.slice(9))}` : null;
7374useEffect(() => {
234setLoading(true);
235try {
236const response = await fetch(`/api/blobs?prefix=${encodeKey(searchPrefix)}&limit=${limit}`);
237const data = await response.json();
238setBlobs(data);
261setBlobContentLoading(true);
262try {
263const response = await fetch(`/api/blob?key=${encodeKey(clickedBlob.key)}`);
264const content = await response.text();
265setSelectedBlob({ ...clickedBlob, key: decodeKey(clickedBlob.key) });
275const handleSave = async () => {
276try {
277await fetch(`/api/blob?key=${encodeKey(selectedBlob.key)}`, {
278method: "PUT",
279body: editContent,
287const handleDelete = async (key) => {
288try {
289await fetch(`/api/blob?key=${encodeKey(key)}`, { method: "DELETE" });
290setBlobs(blobs.filter(b => b.key !== key));
291if (selectedBlob && selectedBlob.key === key) {
304const key = `${searchPrefix}${file.name}`;
305formData.append("key", encodeKey(key));
306await fetch("/api/blob", { method: "POST", body: formData });
307const newBlob = { key, size: file.size, lastModified: new Date().toISOString() };
308setBlobs([newBlob, ...blobs]);
326try {
327const fullKey = `${searchPrefix}${key}`;
328await fetch(`/api/blob?key=${encodeKey(fullKey)}`, {
329method: "PUT",
330body: "",
341const handleDownload = async (key) => {
342try {
343const response = await fetch(`/api/blob?key=${encodeKey(key)}`);
344const blob = await response.blob();
345const url = window.URL.createObjectURL(blob);
360if (newKey && newKey !== oldKey) {
361try {
362const response = await fetch(`/api/blob?key=${encodeKey(oldKey)}`);
363const content = await response.blob();
364await fetch(`/api/blob?key=${encodeKey(newKey)}`, {
365method: "PUT",
366body: content,
367});
368await fetch(`/api/blob?key=${encodeKey(oldKey)}`, { method: "DELETE" });
369setBlobs(blobs.map(b => b.key === oldKey ? { ...b, key: newKey } : b));
370if (selectedBlob && selectedBlob.key === oldKey) {
380const newKey = `__public/${key}`;
381try {
382const response = await fetch(`/api/blob?key=${encodeKey(key)}`);
383const content = await response.blob();
384await fetch(`/api/blob?key=${encodeKey(newKey)}`, {
385method: "PUT",
386body: content,
387});
388await fetch(`/api/blob?key=${encodeKey(key)}`, { method: "DELETE" });
389setBlobs(blobs.map(b => b.key === key ? { ...b, key: newKey } : b));
390if (selectedBlob && selectedBlob.key === key) {
399const newKey = key.slice(9); // Remove "__public/" prefix
400try {
401const response = await fetch(`/api/blob?key=${encodeKey(key)}`);
402const content = await response.blob();
403await fetch(`/api/blob?key=${encodeKey(newKey)}`, {
404method: "PUT",
405body: content,
406});
407await fetch(`/api/blob?key=${encodeKey(key)}`, { method: "DELETE" });
408setBlobs(blobs.map(b => b.key === key ? { ...b, key: newKey } : b));
409if (selectedBlob && selectedBlob.key === key) {
554onClick={() =>
555copyToClipboard(
556`${window.location.origin}/api/public/${encodeURIComponent(selectedBlob.key.slice(9))}`,
557)}
558className="text-blue-400 hover:text-blue-300 text-sm"
577>
578<img
579src={`/api/blob?key=${encodeKey(selectedBlob.key)}`}
580alt="Blob content"
581className="max-w-full h-auto"
e424b5getEdgarFilings1 match
109110if (!response.ok) {
111throw new Error(`SEC API error: ${response.status} ${response.statusText}`);
112}
113
stevensDemoREADME.md5 matches
8## Hono
910This app uses [Hono](https://hono.dev/) as the API framework. You can think of Hono as a replacement for [ExpressJS](https://expressjs.com/) that works in serverless environments like Val Town or Cloudflare Workers. If you come from Python or Ruby, Hono is also a lot like [Flask](https://github.com/pallets/flask) or [Sinatra](https://github.com/sinatra/sinatra), respectively.
1112## Serving assets to the frontend
20### `index.html`
2122The most complicated part of this backend API is serving index.html. In this app (like most apps) we serve it at the root, ie `GET /`.
2324We *bootstrap* `index.html` with some initial data from the server, so that it gets dynamically injected JSON data without having to make another round-trip request to the server to get that data on the frontend. This is a common pattern for client-side rendered apps.
2526## CRUD API Routes
2728This app has two CRUD API routes: for reading and inserting into the messages table. They both speak JSON, which is standard. They import their functions from `/backend/database/queries.ts`. These routes are called from the React app to refresh and update data.
2930## Errors
3132Hono and other API frameworks have a habit of swallowing up Errors. We turn off this default behavior by re-throwing errors, because we think most of the time you'll want to see the full stack trace instead of merely "Internal Server Error". You can customize how you want errors to appear.
FarcasterSpacesHome.tsx2 matches
3738function Spaces() {
39const queryFn = () => fetch(`/api/channels`).then(r => r.json()).then(r => r?.data?.channels);
40const { data: spaces } = useQuery({ queryKey: ["spaces"], queryFn });
41console.log(spaces);
88onChange={e => setChannel(e.target.value)}
89placeholder="Space Title"
90autoCapitalize="on"
91autoCorrect="on"
92type="text"
FarcasterSpacesneynar.ts2 matches
1const baseUrl = "/neynar-proxy?path=";
2// const baseUrl = "https://api.neynar.com/v2/farcaster/";
34export async function fetchNeynarGet(path: string) {
8"Content-Type": "application/json",
9"x-neynar-experimental": "true",
10"x-api-key": "NEYNAR_API_DOCS",
11},
12});
FarcasterSpacesneynar.ts4 matches
1const NEYNAR_API_KEY = Deno.env.get("NEYNAR_API_KEY") || "NEYNAR_API_DOCS";
2const headers = {
3"Content-Type": "application/json",
4"x-api-key": NEYNAR_API_KEY,
5};
67export const fetchGet = async (path: string) => {
8return await fetch("https://api.neynar.com/v2/farcaster/" + path, {
9method: "GET",
10headers: headers,
1314export const fetchPost = async (path: string, body: any) => {
15return await fetch("https://api.neynar.com/v2/farcaster/" + path, {
16method: "POST",
17headers: headers,
14## Discover the profound understandings of a philosopher who challenged humanity to break free from all conditioning.
1516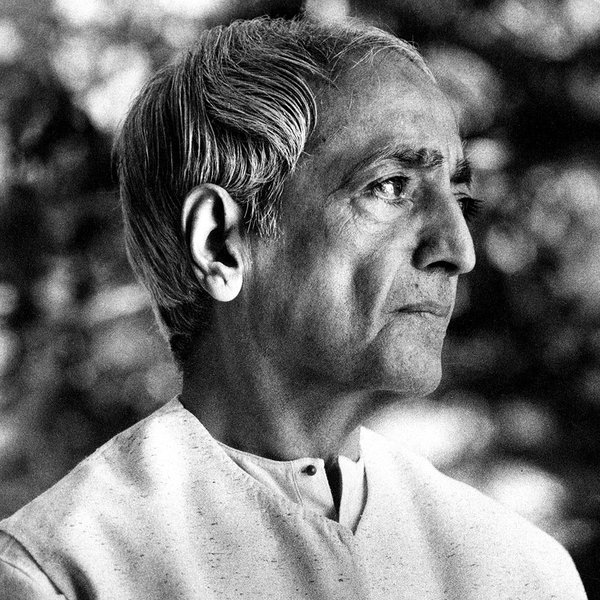
1718*Jiddu Krishnamurti, a philosopher whose teachings emphasized direct perception and freedom.*
26---
2728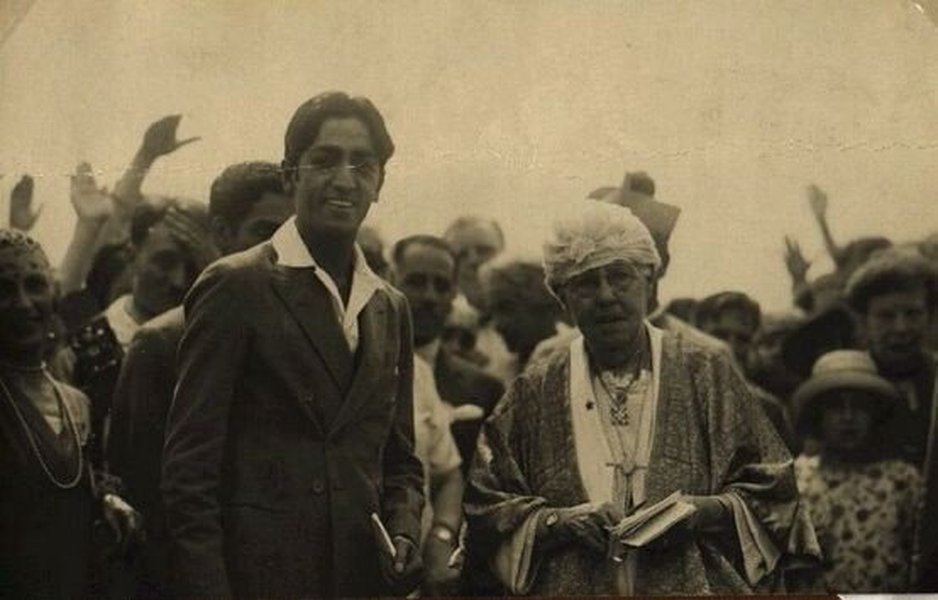
2930*Krishnamurti (right) during his early years with his mentor Annie Besant (left), a key figure in the Theosophical Society.*
47* **Immediacy of Truth:** Truth is not a distant goal to be achieved through time or practice, but a living reality to be perceived in the present moment, free from the distortions of the past or projections of the future.
4849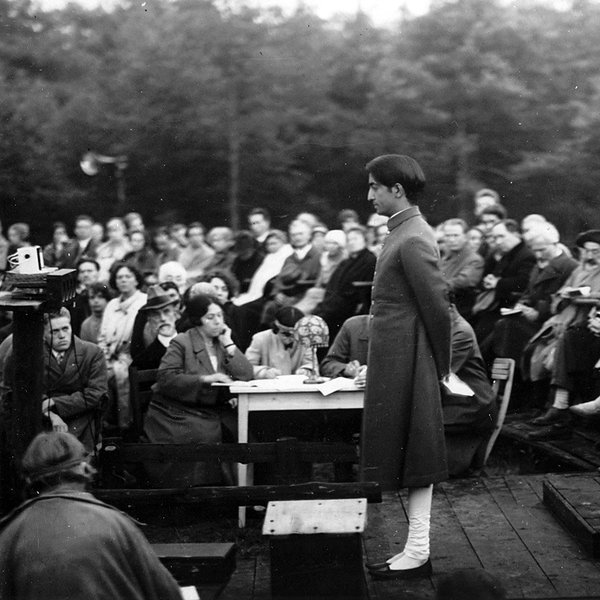
5051*A visual representation related to Krishnamurti's historic 1929 speech where he declared "Truth is a pathless land."*
176The goal was not merely academic excellence but the emergence of mature, sensitive, intelligent human beings capable of living peacefully and creatively in a complex world.
177178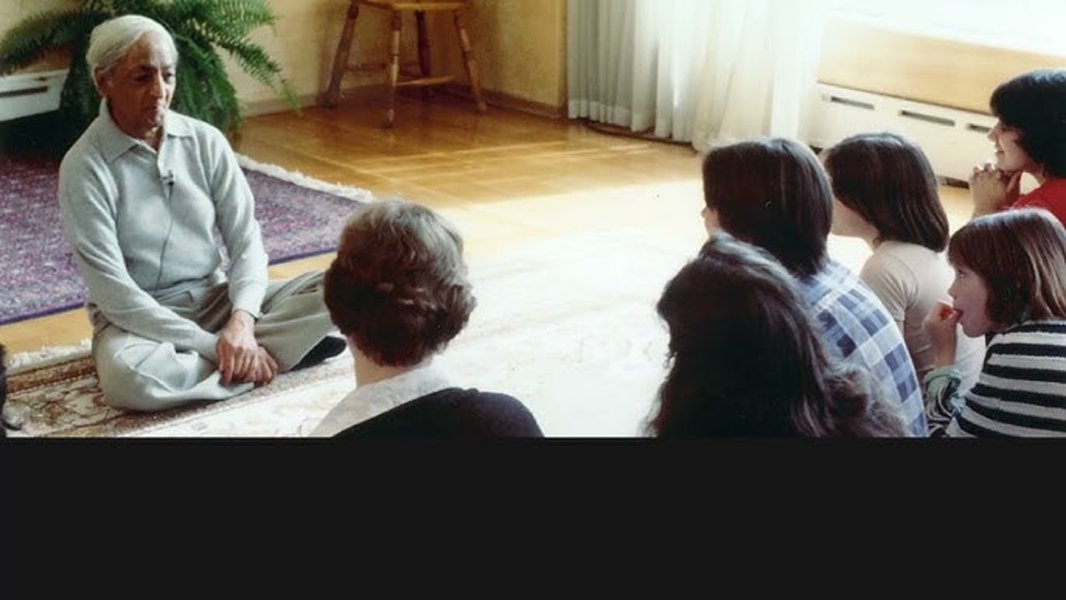
179180*Krishnamurti engaging in discussion, reflecting his emphasis on dialogue and inquiry in education.*
MyPersonalBotsendDailyBrief.ts8 matches
9798export async function sendDailyBriefing(chatId?: string, today?: DateTime) {
99// Get API keys from environment
100const apiKey = Deno.env.get("ANTHROPIC_API_KEY");
101const telegramToken = Deno.env.get("TELEGRAM_TOKEN");
102106}
107108if (!apiKey) {
109console.error("Anthropic API key is not configured.");
110return;
111}
122123// Initialize Anthropic client
124const anthropic = new Anthropic({ apiKey });
125126// Initialize Telegram bot
162163// disabled title for now, it seemes unnecessary...
164// await bot.api.sendMessage(chatId, `*${title}*`, { parse_mode: "Markdown" });
165166// Then send the main content
169170if (content.length <= MAX_LENGTH) {
171await bot.api.sendMessage(chatId, content, { parse_mode: "Markdown" });
172// Store the briefing in chat history
173await storeChatMessage(
198// Send each chunk as a separate message and store in chat history
199for (const chunk of chunks) {
200await bot.api.sendMessage(chatId, chunk, { parse_mode: "Markdown" });
201// Store each chunk in chat history
202await storeChatMessage(