7function App() {
8 const [prompt, setPrompt] = useState("");
9 const [imageUrl, setImageUrl] = useState("");
10 const [loading, setLoading] = useState(false);
11
12 const generateImage = async (e?: React.FormEvent) => {
13 e?.preventDefault();
14 setLoading(true);
21 input: {
22 prompt,
23 image_size: "landscape_4_3",
24 num_inference_steps: 4,
25 num_images: 1,
26 enable_safety_checker: true,
27 sync_mode: true,
28 },
29 });
30 setImageUrl(result.data.images[0].url);
31 } catch (error) {
32 console.error("Error generating image:", error);
33 } finally {
34 setLoading(false);
39 <div className="min-h-screen bg-black text-white py-12 px-4 sm:px-6 lg:px-8">
40 <div className="max-w-3xl mx-auto">
41 <h1 className="text-4xl font-bold text-center mb-8">Fal AI Image Generator</h1>
42 <div className="bg-gray-900 rounded-lg p-6 mb-8 shadow-lg">
43 <form className="flex flex-col sm:flex-row gap-4" onSubmit={generateImage}>
44 <input
45 type="text"
46 value={prompt}
47 onChange={(e) => setPrompt(e.target.value)}
48 placeholder="Enter your image prompt"
49 className="flex-grow px-4 py-2 bg-gray-800 border border-gray-700 rounded-md focus:ring-2 focus:ring-blue-500 focus:border-transparent"
50 />
51 <button
52 onClick={generateImage}
53 disabled={loading || !prompt}
54 className={`px-6 py-2 rounded-md text-white font-medium transition-colors duration-200 ${
80 )
81 : (
82 "Generate Image"
83 )}
84 </button>
85 </form>
86 </div>
87 {imageUrl && (
88 <div className="bg-gray-900 rounded-lg overflow-hidden shadow-lg">
89 <img src={imageUrl} alt="Generated image" className="w-full h-auto" />
90 </div>
91 )}
118 <meta charset="UTF-8">
119 <meta name="viewport" content="width=device-width, initial-scale=1.0">
120 <title>Fal AI Image Generator</title>
121 <script src="https://cdn.tailwindcss.com"></script>
122 <style>
18 const questions = $("[class*='question_content_']");
19 const htmlContent = questions.html();
20 const images = questions.find("img");
21
22 for (const image of images.toArray()) {
23 const src = $(image).attr("src"); // Use Cheerio to get the src attribute
24
25 // Check if src contains any of the specified strings
29
30 // Update the src attribute
31 $(image).attr("src", `https://archive.cool.com/images/${encodeURIComponent(src)}`);
32 }
33
7function App() {
8 const [prompt, setPrompt] = useState("");
9 const [imageUrl, setImageUrl] = useState("");
10 const [loading, setLoading] = useState(false);
11
12 const generateImage = async (e?: React.FormEvent) => {
13 e?.preventDefault();
14 setLoading(true);
21 input: {
22 prompt,
23 image_size: "landscape_4_3",
24 num_inference_steps: 4,
25 num_images: 1,
26 enable_safety_checker: true,
27 sync_mode: true,
28 },
29 });
30 setImageUrl(result.data.images[0].url);
31 } catch (error) {
32 console.error("Error generating image:", error);
33 } finally {
34 setLoading(false);
39 <div className="min-h-screen bg-black text-white py-12 px-4 sm:px-6 lg:px-8">
40 <div className="max-w-3xl mx-auto">
41 <h1 className="text-4xl font-bold text-center mb-8">Fal AI Image Generator</h1>
42 <div className="bg-gray-900 rounded-lg p-6 mb-8 shadow-lg">
43 <form className="flex flex-col sm:flex-row gap-4" onSubmit={generateImage}>
44 <input
45 type="text"
46 value={prompt}
47 onChange={(e) => setPrompt(e.target.value)}
48 placeholder="Enter your image prompt"
49 className="flex-grow px-4 py-2 bg-gray-800 border border-gray-700 rounded-md focus:ring-2 focus:ring-blue-500 focus:border-transparent"
50 />
51 <button
52 onClick={generateImage}
53 disabled={loading || !prompt}
54 className={`px-6 py-2 rounded-md text-white font-medium transition-colors duration-200 ${
80 )
81 : (
82 "Generate Image"
83 )}
84 </button>
85 </form>
86 </div>
87 {imageUrl && (
88 <div className="bg-gray-900 rounded-lg overflow-hidden shadow-lg">
89 <img src={imageUrl} alt="Generated image" className="w-full h-auto" />
90 </div>
91 )}
118 <meta charset="UTF-8">
119 <meta name="viewport" content="width=device-width, initial-scale=1.0">
120 <title>Fal AI Image Generator</title>
121 <script src="https://cdn.tailwindcss.com"></script>
122 <style>
7 Follow,
8 generateCryptoKeyPair,
9 Image,
10 importJwk,
11 LanguageString,
55 assertionMethods: keyPairs.map(pair => pair.multikey),
56 url: new URL("/", ctx.url),
57 icon: new Image({
58 mediaType: "image/png",
59 url: new URL("https://fedify.dev/logo.png"),
60 }),
3This is a lightweight Blob Admin interface to view and debug your Blob data.
4
5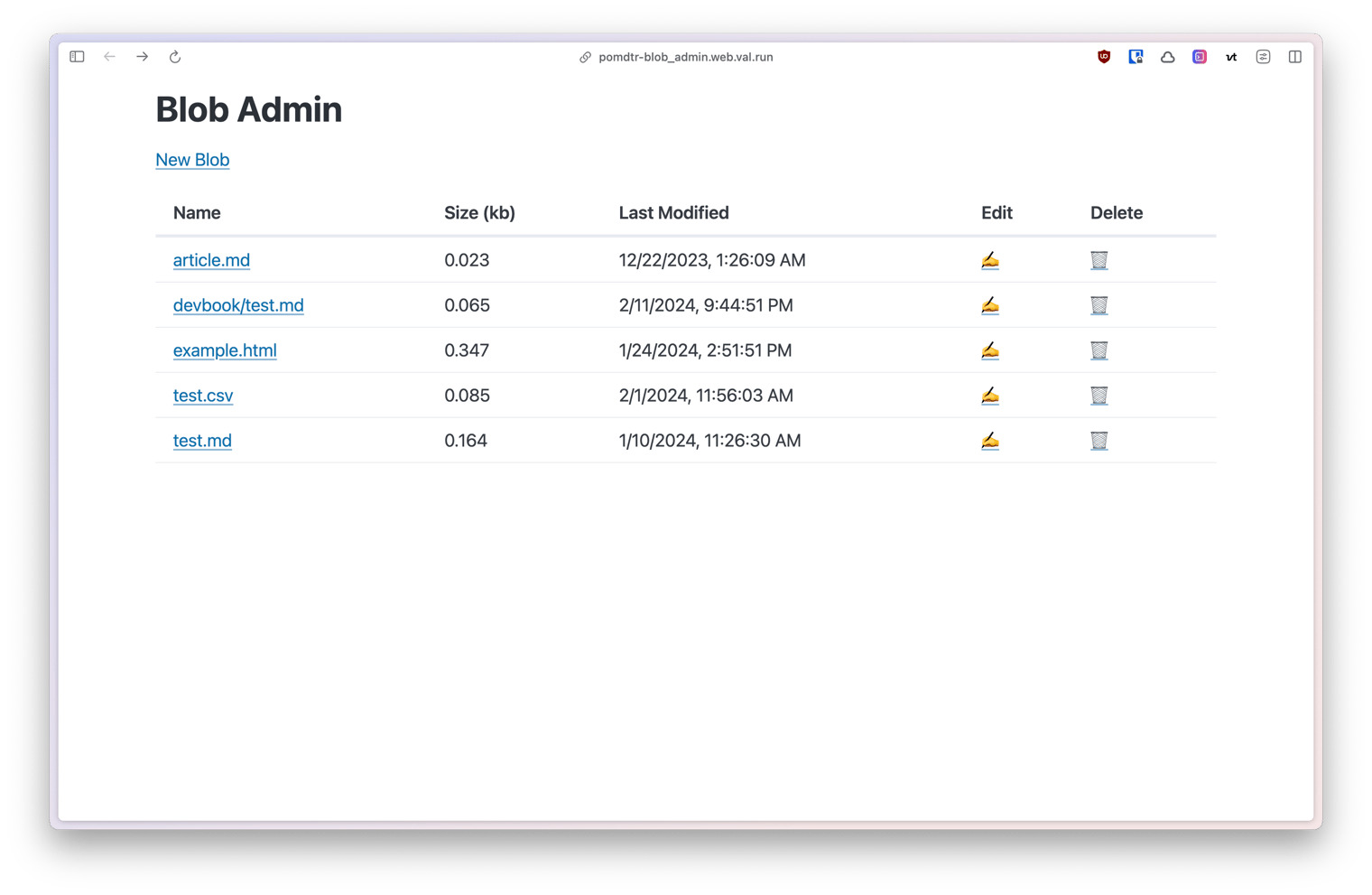
6
7## Installation
47 <div className="header">
48 <h1>Solana Transaction Code Generator</h1>
49 <img src="https://i.postimg.cc/SQV1FgkV/manlet.jpg" alt="Solana Meme" className="meme-image" />
50 </div>
51 <form onSubmit={handleSubmit}>
190 padding: 0;
191 background-color: var(--solana-black);
192 background-image: linear-gradient(45deg, #14F195 25%, #9945FF 25%, #9945FF 50%, #14F195 50%, #14F195 75%, #9945FF 75%, #9945FF 100%);
193 background-size: 56.57px 56.57px;
194 min-height: 100vh;
218}
219
220.meme-image {
221 width: 100px;
222 height: 100px;
7function App() {
8 const [prompt, setPrompt] = useState("");
9 const [imageUrl, setImageUrl] = useState("");
10 const [loading, setLoading] = useState(false);
11
12 const generateImage = async (e?: React.FormEvent) => {
13 e?.preventDefault();
14 setLoading(true);
21 input: {
22 prompt,
23 image_size: "landscape_4_3",
24 num_inference_steps: 4,
25 num_images: 1,
26 enable_safety_checker: true,
27 sync_mode: true,
28 },
29 });
30 setImageUrl(result.data.images[0].url);
31 } catch (error) {
32 console.error("Error generating image:", error);
33 } finally {
34 setLoading(false);
39 <div className="min-h-screen bg-black text-white py-12 px-4 sm:px-6 lg:px-8">
40 <div className="max-w-3xl mx-auto">
41 <h1 className="text-4xl font-bold text-center mb-8">Fal AI Image Generator</h1>
42 <div className="bg-gray-900 rounded-lg p-6 mb-8 shadow-lg">
43 <form className="flex flex-col sm:flex-row gap-4" onSubmit={generateImage}>
44 <input
45 type="text"
46 value={prompt}
47 onChange={(e) => setPrompt(e.target.value)}
48 placeholder="Enter your image prompt"
49 className="flex-grow px-4 py-2 bg-gray-800 border border-gray-700 rounded-md focus:ring-2 focus:ring-blue-500 focus:border-transparent"
50 />
51 <button
52 onClick={generateImage}
53 disabled={loading || !prompt}
54 className={`px-6 py-2 rounded-md text-white font-medium transition-colors duration-200 ${
80 )
81 : (
82 "Generate Image"
83 )}
84 </button>
85 </form>
86 </div>
87 {imageUrl && (
88 <div className="bg-gray-900 rounded-lg overflow-hidden shadow-lg">
89 <img src={imageUrl} alt="Generated image" className="w-full h-auto" />
90 </div>
91 )}
118 <meta charset="UTF-8">
119 <meta name="viewport" content="width=device-width, initial-scale=1.0">
120 <title>Fal AI Image Generator</title>
121 <script src="https://cdn.tailwindcss.com"></script>
122 <style>
7function App() {
8 const [prompt, setPrompt] = useState("");
9 const [imageUrl, setImageUrl] = useState("");
10 const [loading, setLoading] = useState(false);
11
12 const generateImage = async (e?: React.FormEvent) => {
13 e?.preventDefault();
14 setLoading(true);
21 input: {
22 prompt,
23 image_size: "landscape_4_3",
24 num_inference_steps: 4,
25 num_images: 1,
26 enable_safety_checker: true,
27 sync_mode: true,
28 },
29 });
30 setImageUrl(result.data.images[0].url);
31 } catch (error) {
32 console.error("Error generating image:", error);
33 } finally {
34 setLoading(false);
39 <div className="min-h-screen bg-black text-white py-12 px-4 sm:px-6 lg:px-8">
40 <div className="max-w-3xl mx-auto">
41 <h1 className="text-4xl font-bold text-center mb-8">Fal AI Image Generator</h1>
42 <div className="bg-gray-900 rounded-lg p-6 mb-8 shadow-lg">
43 <form className="flex flex-col sm:flex-row gap-4" onSubmit={generateImage}>
44 <input
45 type="text"
46 value={prompt}
47 onChange={(e) => setPrompt(e.target.value)}
48 placeholder="Enter your image prompt"
49 className="flex-grow px-4 py-2 bg-gray-800 border border-gray-700 rounded-md focus:ring-2 focus:ring-blue-500 focus:border-transparent"
50 />
51 <button
52 onClick={generateImage}
53 disabled={loading || !prompt}
54 className={`px-6 py-2 rounded-md text-white font-medium transition-colors duration-200 ${
80 )
81 : (
82 "Generate Image"
83 )}
84 </button>
85 </form>
86 </div>
87 {imageUrl && (
88 <div className="bg-gray-900 rounded-lg overflow-hidden shadow-lg">
89 <img src={imageUrl} alt="Generated image" className="w-full h-auto" />
90 </div>
91 )}
118 <meta charset="UTF-8">
119 <meta name="viewport" content="width=device-width, initial-scale=1.0">
120 <title>Fal AI Image Generator</title>
121 <script src="https://cdn.tailwindcss.com"></script>
122 <style>
17 "settings": {
18 "url": url,
19 "optionStr": "content,metadata,screenshot,pageshot,citation,doi,images,links",
20 "crawlMode": "jina",
21 "outputMode": "json"
188 title: '',
189 description: '',
190 image: '',
191 pageshotUrl: '',
192 screenshotUrl: ''
323 const title = metadata.title || metadata['og:title'] || metadata['twitter:title'] || 'No title available';
324 const description = metadata.description || metadata['og:description'] || metadata['twitter:description'] || 'No description available';
325 const image = metadata['og:image'] || metadata['twitter:image'] || '';
326 const pageshotUrl = result.jina?.pageshot || '';
327 const screenshotUrl = result.jina?.screenshot || '';
333 title,
334 description,
335 image,
336 pageshotUrl: result.jina?.pageshot,
337 screenshotUrl: result.jina?.screenshot
392 <meta charset="UTF-8">
393 <meta name="viewport" content="width=device-width, initial-scale=1.0">
394 <link rel="icon" type="image/png" href="https://labspace.ai/ls2-circle.png" />
395 <title>PageShot — Get data about any web page</title>
396 <meta property="og:title" content="PageShot — Get data about any web page" />
397 <meta property="og:description" content="Get data about any web page" />
398 <meta property="og:image" content="https://yawnxyz-og.web.val.run/img?link=https://pageshot.labspace.ai&title=PageShot&subtitle=Get+data+about+any+web+page" />
399 <meta property="og:url" content="https://pageshot.labspace.ai" />
400 <meta property="og:type" content="website" />
401 <meta name="twitter:card" content="summary_large_image" />
402 <meta name="twitter:title" content="PageShot — Get data about any web page" />
403 <meta name="twitter:description" content="Get data about any web page" />
404 <meta name="twitter:image" content="https://yawnxyz-og.web.val.run/img?link=https://pageshot.labspace.ai&title=PageShot&subtitle=Get+data+about+any+web+page" />
405 <script src="https://cdn.tailwindcss.com"></script>
406 <script src="https://unpkg.com/dexie@3.2.2/dist/dexie.js"></script>
439 <div class="OutputHeader bg-app-bg-alt p-4 rounded-lg mb-4">
440 <div class="rounded-lg mb-4" x-show="metadata.title">
441 <template x-if="metadata.image">
442 <img :src="metadata.image.startsWith('http') ? metadata.image : new URL(metadata.image, input).href"
443 alt="Page preview"
444 class="max-w-48 h-auto rounded mt-2 mb-4">
7function App() {
8 const [prompt, setPrompt] = useState("");
9 const [imageUrl, setImageUrl] = useState("");
10 const [loading, setLoading] = useState(false);
11
12 const generateImage = async (e?: React.FormEvent) => {
13 e?.preventDefault();
14 setLoading(true);
21 input: {
22 prompt,
23 image_size: "landscape_4_3",
24 num_inference_steps: 4,
25 num_images: 1,
26 enable_safety_checker: true,
27 sync_mode: true,
28 },
29 });
30 setImageUrl(result.data.images[0].url);
31 } catch (error) {
32 console.error("Error generating image:", error);
33 } finally {
34 setLoading(false);
39 <div className="min-h-screen bg-black text-white py-12 px-4 sm:px-6 lg:px-8">
40 <div className="max-w-3xl mx-auto">
41 <h1 className="text-4xl font-bold text-center mb-8">Fal AI Image Generator</h1>
42 <div className="bg-gray-900 rounded-lg p-6 mb-8 shadow-lg">
43 <form className="flex flex-col sm:flex-row gap-4" onSubmit={generateImage}>
44 <input
45 type="text"
46 value={prompt}
47 onChange={(e) => setPrompt(e.target.value)}
48 placeholder="Enter your image prompt"
49 className="flex-grow px-4 py-2 bg-gray-800 border border-gray-700 rounded-md focus:ring-2 focus:ring-blue-500 focus:border-transparent"
50 />
51 <button
52 onClick={generateImage}
53 disabled={loading || !prompt}
54 className={`px-6 py-2 rounded-md text-white font-medium transition-colors duration-200 ${
80 )
81 : (
82 "Generate Image"
83 )}
84 </button>
85 </form>
86 </div>
87 {imageUrl && (
88 <div className="bg-gray-900 rounded-lg overflow-hidden shadow-lg">
89 <img src={imageUrl} alt="Generated image" className="w-full h-auto" />
90 </div>
91 )}
118 <meta charset="UTF-8">
119 <meta name="viewport" content="width=device-width, initial-scale=1.0">
120 <title>Fal AI Image Generator</title>
121 <script src="https://cdn.tailwindcss.com"></script>
122 <style>