getJsonAndRenderAsImageREADME.md13 matches
1# getJsonAndRenderAsImage
23Shows how to get a JSON object containing a base64 imaged stored in Val Town blob storage and render it as an image in the DOM with React.
45## Setup
67Make sure you have a JSON object named `image-test` in your Val Town blob storage (alternatively, you can change the key name in the blob getter in the script).
89The object should look like this:
11```json
12{
13"image": "base64stringgoeshere"
14}
15```
1617To easily upload an image to your blob storage, [fork this val](
18getBlobAndRenderAsImage), run it, and enter your API key in the password input.
1920## How it works
221. Fetching the JSON:
2324- The client-side React component makes a fetch request to "/image".
25- This request is handled by our server function.
26282. Server-side handling:
2930- The server function calls `blob.getJSON("image-test")`.
31- This `blob.getJSON()` method makes an HTTP request to the Val Town API.
32- The API returns a Response object containing the JSON data.
546. Creating a data URL:
5556- We extract the image data from the JSON object at `data.image`.
57- We prepend the data URL prefix to the image data.
58- This data URL is a base64-encoded string representing the image.
59606465668. Rendering the image:
6768- We use the data URL as the src attribute of an `<img>` tag.
69- The browser decodes the base64 data and renders the image.
7071## Further reading on Val Town blobs
hackerNewsDigestREADME.md1 match
456<img width="400px" src="https://imagedelivery.net/iHX6Ovru0O7AjmyT5yZRoA/2661d748-d7a7-4d1e-85a4-f60fae262000/public" />
7
1011<div align="center">
12<img src="https://imagedelivery.net/iHX6Ovru0O7AjmyT5yZRoA/67a1d35e-c37c-41a4-0e5a-03a9ba585d00/public" width="500px"/>
13</div>
45<div align="center">
6<img src="https://imagedelivery.net/iHX6Ovru0O7AjmyT5yZRoA/67a1d35e-c37c-41a4-0e5a-03a9ba585d00/public" width="700px"/>
7</div>
placeholderKittenImagesmain.tsx28 matches
1// This val creates a publicly accessible kitten image generator using the Val Town image generation API.
2// It supports generating square images with a single dimension parameter or rectangular images with two dimension parameters.
34export default async function server(request: Request): Promise<Response> {
13<meta charset="UTF-8">
14<meta name="viewport" content="width=device-width, initial-scale=1.0">
15<title>Kitten Image Generator</title>
16<style>
17body {
31margin-bottom: 20px;
32}
33.example-image {
34display: block;
35max-width: 100%;
75</head>
76<body>
77<h1>Welcome to the Kitten Image Generator!</h1>
78<div class="instructions">
79<p>Use this service to generate cute kitten images of various sizes:</p>
80<p>For square images: <code>/width</code> (e.g., <code>/400</code> for a 400x400 image)</p>
81<p>For rectangular images: <code>/width/height</code> (e.g., <code>/400/300</code> for a 400x300 image)</p>
82</div>
83<div class="form-container">
84<h2>Generate a Kitten Image</h2>
85<form id="kittenForm">
86<label for="width">Width (64-1024):</label>
91</form>
92</div>
93<p>Here's an example of a generated 400x400 kitten image:</p>
94<img src="/400" alt="Example 400x400 kitten" class="example-image">
95<p>Start generating your own kitten images by using the form above or modifying the URL!</p>
96<script>
97document.getElementById('kittenForm').addEventListener('submit', function(e) {
111112if (parts.length === 1) {
113// Square image
114width = height = parseInt(parts[0]);
115} else if (parts.length === 2) {
116// Rectangular image
117width = parseInt(parts[0]);
118height = parseInt(parts[1]);
119} else {
120return new Response('Invalid URL format. Use /width for square images or /width/height for rectangular images.',
121{ status: 400, headers: { 'Content-Type': 'text/plain' } });
122}
132133const prompt = `A cute kitten, high quality, detailed`;
134const imageGenerationUrl = `https://maxm-imggenurl.web.val.run/${encodeURIComponent(prompt)}?width=${width}&height=${height}`;
135136try {
137console.log(`Generating image with dimensions: ${width}x${height}`);
138const imageResponse = await fetch(imageGenerationUrl);
139console.log(`Response status: ${imageResponse.status}`);
140141if (!imageResponse.ok) {
142throw new Error(`Failed to generate image: ${imageResponse.status} ${imageResponse.statusText}`);
143}
144145const imageBlob = await imageResponse.blob();
146console.log(`Successfully generated image, size: ${imageBlob.size} bytes`);
147148// Return the image as-is, without any processing
149return new Response(imageBlob, {
150headers: {
151'Content-Type': 'image/png',
152'Cache-Control': 'public, max-age=86400',
153},
154});
155} catch (error) {
156console.error('Error generating image:', error.message);
157return new Response(`Failed to generate image: ${error.message}`, { status: 500, headers: { 'Content-Type': 'text/plain' } });
158}
159}
getBlobAndRenderAsImageREADME.md11 matches
1# getBlobAndRenderAsImage
23Shows how to get a Val Town blob and render it as an image in the DOM with React.
45## Setup
67Make sure you have an image named `test.png` in your Val Town blob storage (alternatively, you can change the key name in the blob getter in the script).
89To easily upload an image to your blob storage, [fork this val](
10getBlobAndRenderAsImage), run it, and enter your API key in the password input.
1112## How it works
141. Fetching the blob:
1516- The client-side React component makes a fetch request to "/image".
17- This request is handled by our server function.
1822- The server function calls `blob.get("test.png")`.
23- This `blob.get()` method makes an HTTP request to the Val Town API.
24- The API returns a Response object containing the image data.
2526344. Sending the response to the client:
3536- We set the appropriate "Content-Type" header (e.g., "image/png").
37- We return the Response object to the client.
3848- We create a FileReader object.
49- We use FileReader to read the Blob as a data URL.
50- This data URL is a base64-encoded string representing the image.
51525657588. Rendering the image:
5960- We use the data URL as the src attribute of an `<img>` tag.
61- The browser decodes the base64 data and renders the image.
6263## Further reading on Val Town blobs
blob_adminREADME.md1 match
3This is a lightweight Blob Admin interface to view and debug your Blob data.
45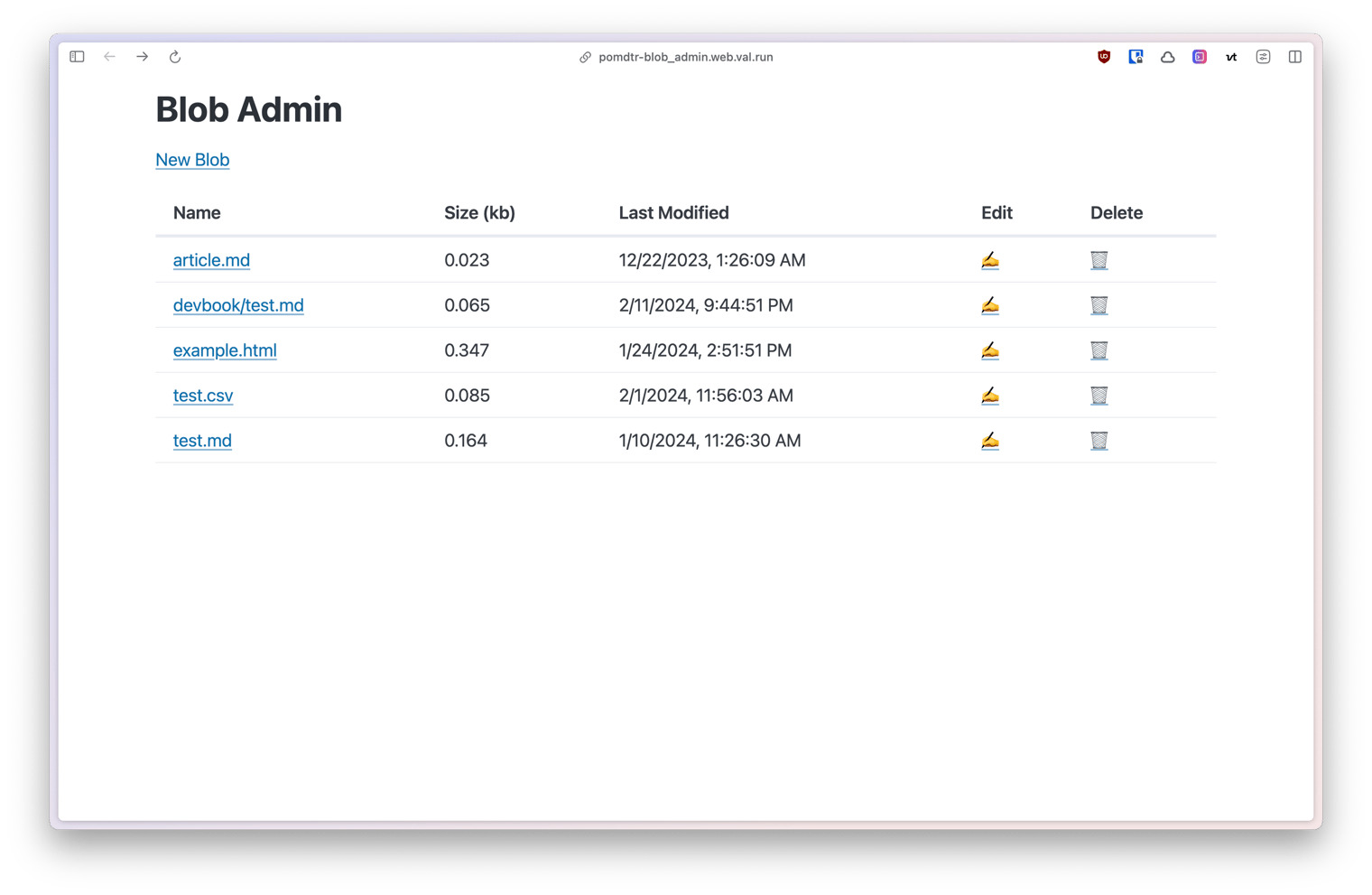
67Use this button to install the val:
ablePinkDogREADME.md1 match
3Protect your vals behind a password. Use session cookies to persist authentication.
45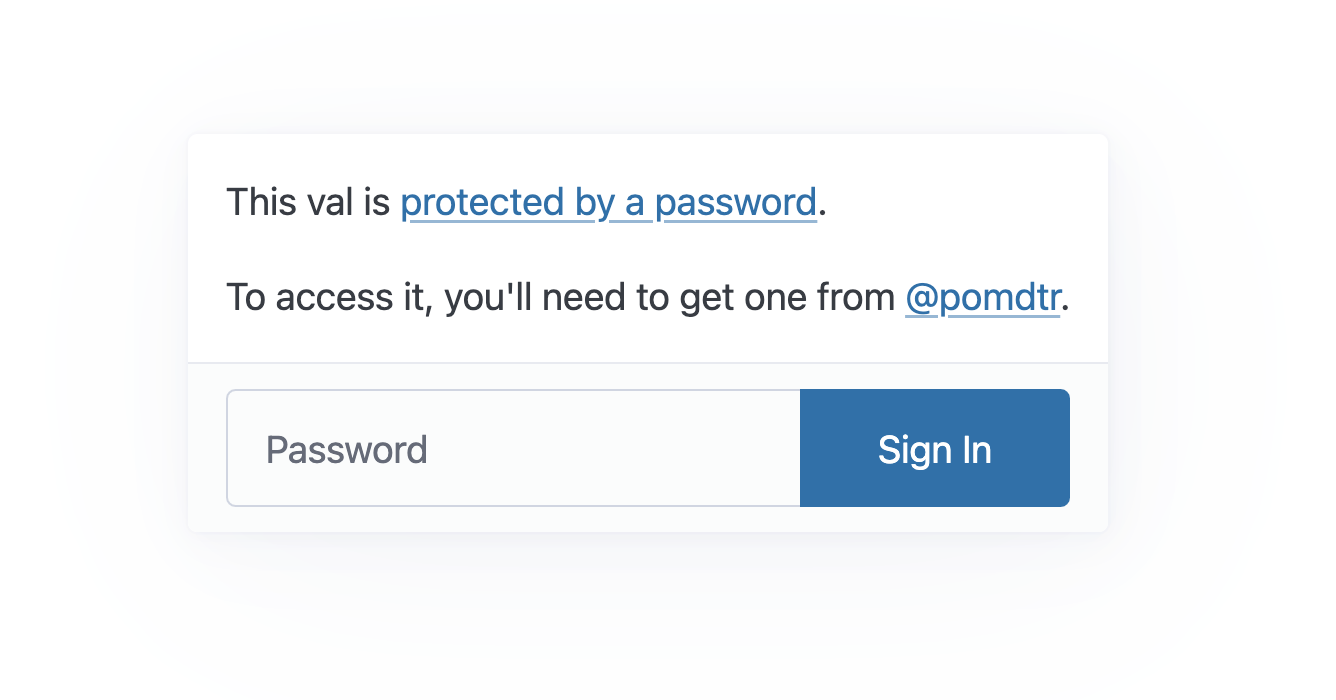
67## Demo
3This is a lightweight Blob Admin interface to view and debug your Blob data.
45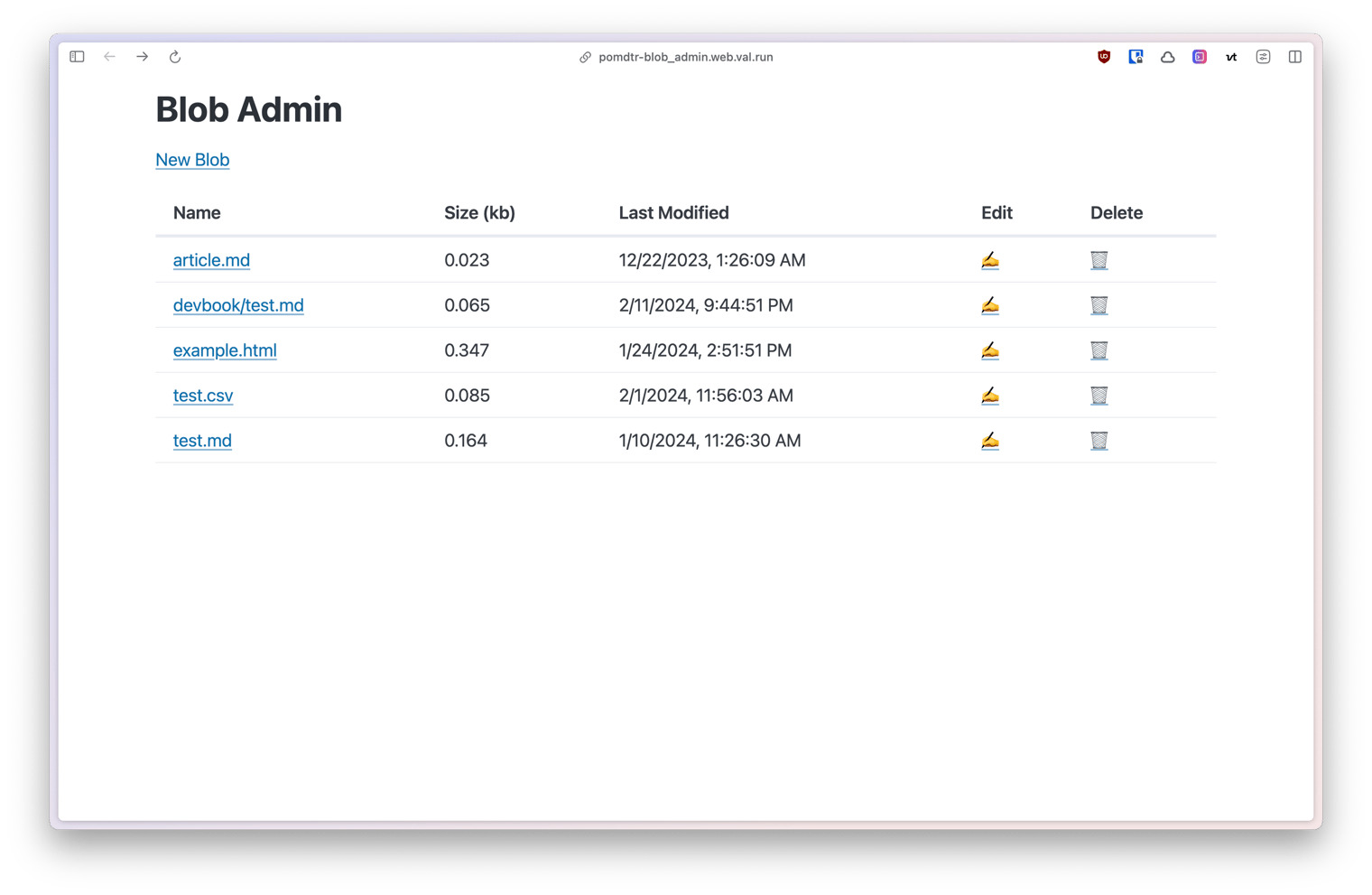
67Use this button to install the val:
3This is a lightweight Blob Admin interface to view and debug your Blob data.
45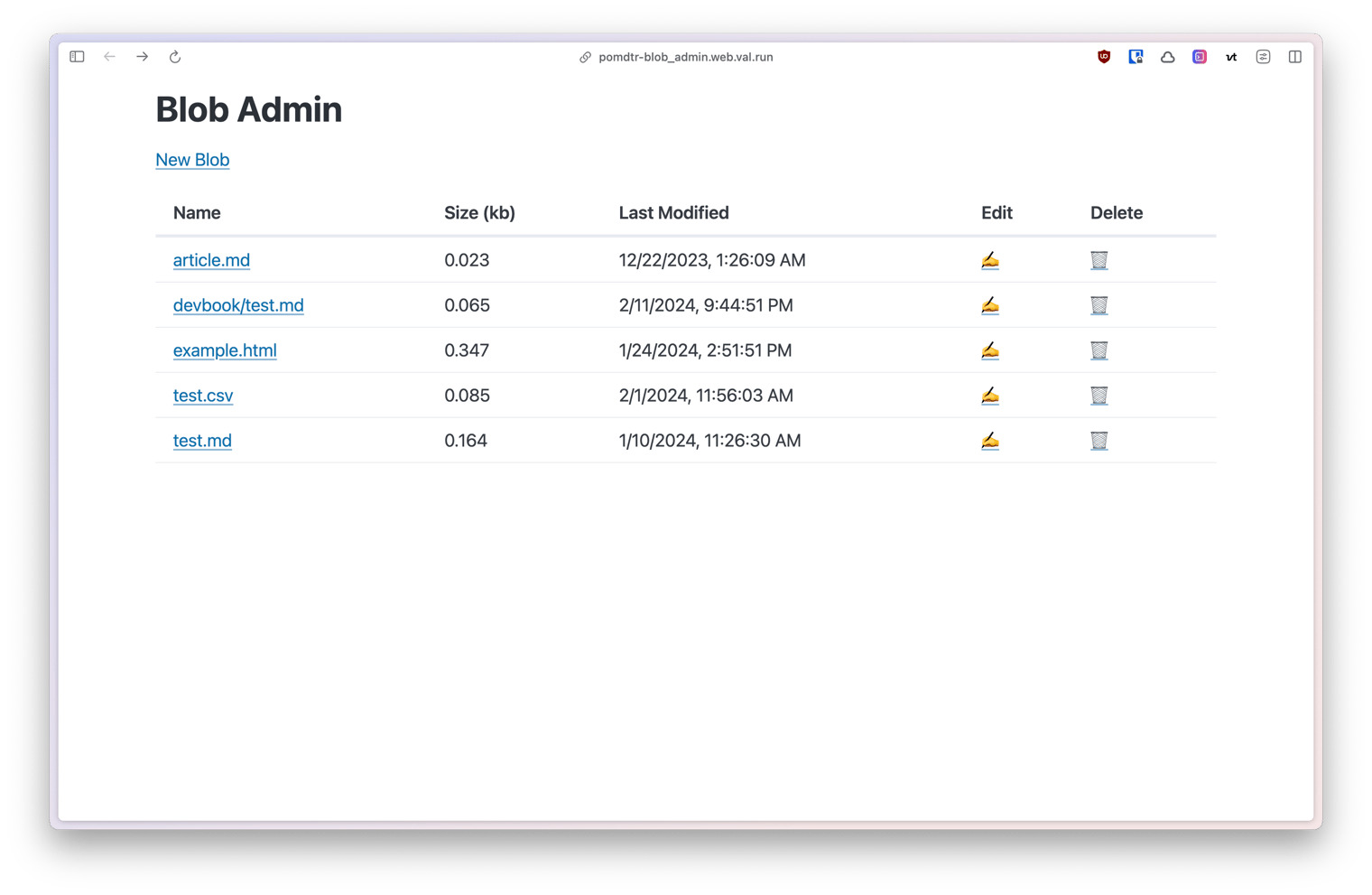
67Use this button to install the val: