11function App() {
12 const [json, setJson] = useState("");
13 const [imageUrl, setImageUrl] = useState("");
14 const [error, setError] = useState("");
15 const [isLoading, setIsLoading] = useState(false);
19 setIsLoading(true);
20 setError("");
21 setImageUrl("");
22
23 try {
32 if (data.error) {
33 setError(data.error);
34 } else if (data.imageUrl) {
35 setImageUrl(data.imageUrl);
36 }
37 } catch (error) {
44 return (
45 <div className="container">
46 <h1>JSON to DALL-E Image Generator</h1>
47 <form onSubmit={handleSubmit}>
48 <textarea
54 >
55 </textarea>
56 <button type="submit" disabled={isLoading}>Generate Image</button>
57 </form>
58 {isLoading && <div id="loadingIndicator">Generating image...</div>}
59 {error && (
60 <div>
62 </div>
63 )}
64 {imageUrl && (
65 <div>
66 <img src={imageUrl} alt="Generated image" />
67 </div>
68 )}
116 font-style: italic;
117 }
118 #imageContainer {
119 margin-top: 20px;
120 }
167 const dallePrompt = completion.choices[0].message.content;
168
169 // Generate DALL-E image
170 const response = await openai.images.generate({
171 model: "dall-e-3",
172 prompt: dallePrompt,
175 });
176
177 return new Response(JSON.stringify({ imageUrl: response.data[0].url, dallePrompt }), {
178 headers: { "Content-Type": "application/json" },
179 });
190 <html>
191 <head>
192 <title>JSON to DALL-E Image Generator</title>
193 <style>${css}</style>
194 </head>
1# ☔️ Umbrella reminder if there's rain today
2
3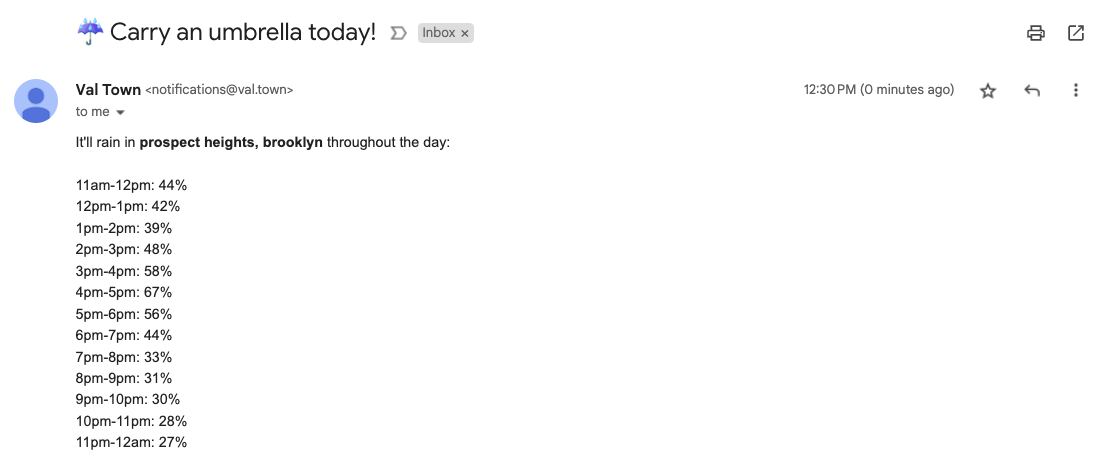
4
5## Setup
4
5
6<img width="400px" src="https://imagedelivery.net/iHX6Ovru0O7AjmyT5yZRoA/2661d748-d7a7-4d1e-85a4-f60fae262000/public" />
7
11 let html = `<h1>${valTownInspo.title}</h1>
12 <p>${valTownInspo.description}</p>
13 <a href="https://val.town/${valTownInspo.val}"><img src="${valTownInspo.image}" style="max-width:576px"/></a>
14 <p><a href="https://www.val.town/settings/intervals">Unsubscribe here</a></p>`;
15
34
35 const dist_nowcast_warnings =
36 "https://reactjs.imd.gov.in/geoserver/imd/wms?service=WMS&request=GetMap&layers=imd%3ANowcast_StateDistrict_Merged&styles=&format=image%2Fsvg&transparent=true&version=1.1.1&width=256&height=256&srs=EPSG%3A4326&bbox="
37 + bbox;
38
39 // env=day:Day1_Color
40 const dist_warnings_today =
41 "https://reactjs.imd.gov.in/geoserver/imd/wms?service=WMS&request=GetMap&layers=imd%3AWarnings_StateDistrict_Merged&styles=&format=image%2Fsvg&transparent=true&version=1.1.1&env=day%3ADay1_Color&width=256&height=256&srs=EPSG%3A4326&bbox="
42 + bbox;
43
44 // env=day:Day2_Color
45 const dist_warnings_today_plus_1 =
46 "https://reactjs.imd.gov.in/geoserver/imd/wms?service=WMS&request=GetMap&layers=imd%3AWarnings_StateDistrict_Merged&styles=&format=image%2Fsvg&transparent=true&version=1.1.1&env=day%3ADay2_Color&width=256&height=256&srs=EPSG%3A4326&bbox="
47 + bbox;
48
49 // env=day:Day3_Color
50 const dist_warnings_today_plus_2 =
51 "https://reactjs.imd.gov.in/geoserver/imd/wms?service=WMS&request=GetMap&layers=imd%3AWarnings_StateDistrict_Merged&styles=&format=image%2Fsvg&transparent=true&version=1.1.1&env=day%3ADay3_Color&width=256&height=256&srs=EPSG%3A4326&bbox="
52 + bbox;
53
54 // env=day:Day4_Color
55 const dist_warnings_today_plus_3 =
56 "https://reactjs.imd.gov.in/geoserver/imd/wms?service=WMS&request=GetMap&layers=imd%3AWarnings_StateDistrict_Merged&styles=&format=image%2Fsvg&transparent=true&version=1.1.1&env=day%3ADay4_Color&width=256&height=256&srs=EPSG%3A4326&bbox="
57 + bbox;
58
59 // env=day:Day5_Color
60 const dist_warnings_today_plus_4 =
61 "https://reactjs.imd.gov.in/geoserver/imd/wms?service=WMS&request=GetMap&layers=imd%3AWarnings_StateDistrict_Merged&styles=&format=image%2Fsvg&transparent=true&version=1.1.1&env=day%3ADay5_Color&width=256&height=256&srs=EPSG%3A4326&bbox="
62 + bbox;
63 /*
4
5<div align="center">
6<img src="https://imagedelivery.net/iHX6Ovru0O7AjmyT5yZRoA/67a1d35e-c37c-41a4-0e5a-03a9ba585d00/public" width="700px"/>
7</div>
218 functions where possible. Unless specified, don't add error handling,
219 make sure that errors bubble up to the caller.
220 Avoid external images or base64 images, use emojis, unicode symtols, or icon fonts/libraries instead, unless that's
221 not practical for the user's request (e.g. if they ask for a particular animated gif).
222 If the user asks for something that requires persistence, use the Val Town Blob storage API, unless
280functions where possible. Unless specified, don't add error handling,
281make sure that errors bubble up to the caller.
282Avoid external images or base64 images, use emojis, unicode symtols, or icon fonts/libraries instead, unless that's
283not practical for the user's request (e.g. if they ask for a particular animated gif).
284If the user asks for something that requires persistence, use the Val Town Blob storage API, unless
315 ---
316
317 Val Town comes with blob storage built-in. It allows for storing any data: text, JSON, images. You can access it via [\`std/blob\`](https://www.val.town/v/std/blob).
318
319 Blob storage is scoped globally to your account. If you set a blob in one val, you can retrieve it by the same key in another val. It's backed by Cloudflare R2.
29 "User-Agent":
30 "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/121.0.0.0 Safari/537.36",
31 "Accept": "text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8",
32 "Accept-Language": "en-US,en;q=0.5",
33 "Sec-Fetch-Site": "cross-site",
3Protect your vals behind a password. Use session cookies to persist authentication.
4
5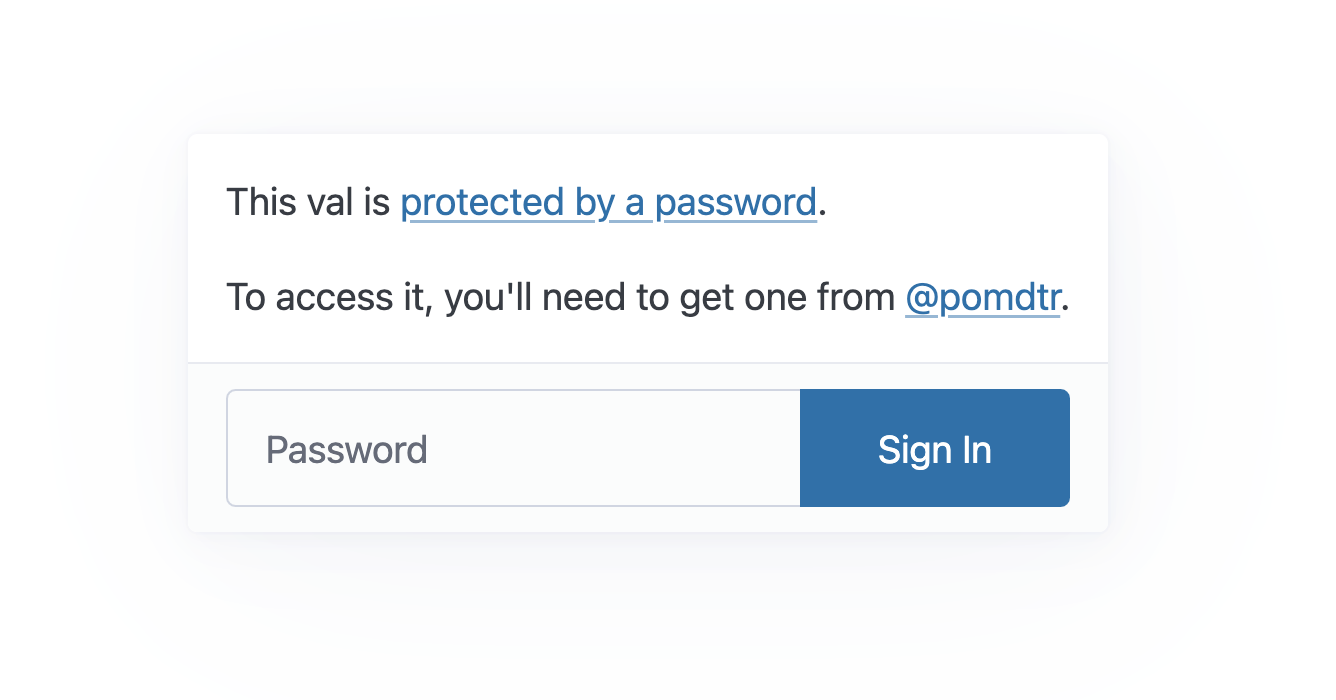
6
7## Demo
31 "highlights": [
32 "Built an event management platform with secure authentication and full CRUD capabilities, streamlining client operations.",
33 "Crafted a website for a food truck that captures their unique branding and playful image. The site features a fun, user-centric interface that facilitates online inquiries and enhances user engagement.",
34 "Designed and developed a responsive, accessibility-focused website for a law firm, improving client accessibility and increasing inquiries by 20%."
35 ]
51 "highlights": [
52 "Enhanced scene consistency with seed settings and introduced controls for motion, quality, and frame rate adjustments.",
53 "Integrated GPT-3.5 for prompt enhancement, significantly improving image and video quality.",
54 "Overcame the challenge of running FFmpeg client-side in Next.js, enabling real-time video processing in the browser."
55 ],