fluxImageGeneratormain.tsx22 matches
1// This app uses the fal.ai API to generate images based on user prompts.
2// It features a clean UI with an input field for the prompt and a button to generate the image.
3// The generated image is displayed below the input field.
4// React is used for the UI and the fal.ai serverless client for image generation.
5// The app measures and displays the latency for each image generation.
6// The background features randomly placed pixelart lightning bolts in neon yellow.
717const BOLT_SIZE = 40; // Width and height of each bolt
18const DEFAULT_PROMPT = "green grass landscape with text \"val.town\" is engraved, a townhouse in the background";
19const PLACEHOLDER_IMAGE = "https://fal.media/files/penguin/PysYf1-_ddhM7JKiLrkcF.png";
20const RATE_LIMIT = 15; // requests per hour
21const RATE_LIMIT_WINDOW = 5 * 60 * 1000; // 5 minutes in milliseconds
72function App() {
73const [prompt, setPrompt] = useState(DEFAULT_PROMPT);
74const [imageUrl, setImageUrl] = useState(PLACEHOLDER_IMAGE);
75const [latency, setLatency] = useState<number | null>(null);
76const [loading, setLoading] = useState(false);
77const [error, setError] = useState("");
7879const generateImage = async () => {
80setLoading(true);
81setError("");
87});
88const data = await response.json();
89if (!response.ok) throw new Error(data.error || "Failed to generate image");
90setLatency(data.latency);
91setImageUrl(data.imageUrl);
92setError(""); // Clear any previous errors
93} catch (err) {
103<Background />
104<div className="container">
105<h1 className="title">AI Image Generator</h1>
106<div className="input-container">
107<input
113/>
114</div>
115<button onClick={generateImage} disabled={loading || !prompt} className="generate-btn">
116{loading ? "Generating..." : "Generate Image"}
117</button>
118{error && <p className="error">{error}</p>}
119<div className="image-container">
120{latency !== null && (
121<p className="latency">Inference time (doesn't include network): {latency.toFixed(2)} ms</p>
123{loading
124? <LoadingSpinner />
125: <img src={imageUrl} alt="Generated image" className="generated-image" />}
126</div>
127<div className="footer">
193input: {
194prompt,
195image_size: "landscape_4_3",
196num_inference_steps: 4,
197num_images: 1,
198enable_safety_checker: true,
199sync_mode: true,
201});
202return new Response(
203JSON.stringify({ imageUrl: result.images[0].url, latency: result.timings.inference * 1000 }),
204{
205headers: { "Content-Type": "application/json" },
207);
208} catch (error) {
209return new Response(JSON.stringify({ error: "Failed to generate image" }), {
210status: 500,
211headers: { "Content-Type": "application/json" },
221<meta charset="UTF-8">
222<meta name="viewport" content="width=device-width, initial-scale=1.0">
223<title>AI Image Generator</title>
224<style>${css}</style>
225</head>
315text-align: center;
316}
317.image-container {
318margin-top: 20px;
319text-align: center;
322border-radius: 10px;
323}
324.generated-image {
325max-width: 100%;
326height: auto;
7* Type text prompts, select it, press "Q". Select a previous generation with a new text prompt to keep iterating. Selecting shapes doesn't work yet. Have fun!
89<a href="https://x.com/JanPaul123/status/1815502582015754657"><img width=500 src="https://imagedelivery.net/iHX6Ovru0O7AjmyT5yZRoA/5893dfbf-c2de-4be0-049e-d8fdd1970a00/public"/></a>
10
10* Create a [Val Town API token](https://www.val.town/settings/api), open the browser preview of this val, and use the API token as the password to log in.
1112<img width=500 src="https://imagedelivery.net/iHX6Ovru0O7AjmyT5yZRoA/7077d1b5-1fa7-4a9b-4b93-f8d01d3e4f00/public"/>
umbrellaReminderREADME.md1 match
1# ☔️ Umbrella reminder if there's rain today
23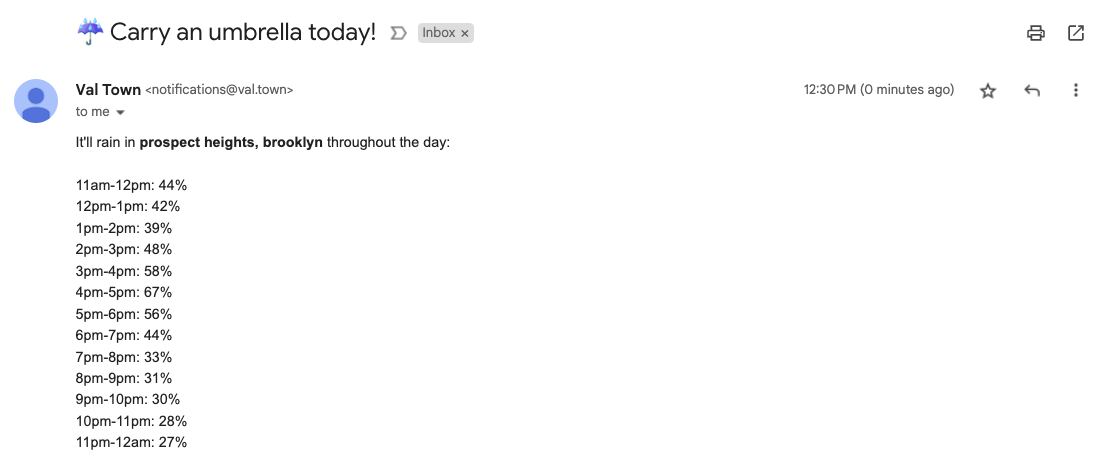
45## Setup
45<div align="center">
6<img src="https://imagedelivery.net/iHX6Ovru0O7AjmyT5yZRoA/67a1d35e-c37c-41a4-0e5a-03a9ba585d00/public" width="700px"/>
7</div>
8
isMyWebsiteDownREADME.md1 match
89<div align="center">
10<img src="https://imagedelivery.net/iHX6Ovru0O7AjmyT5yZRoA/67a1d35e-c37c-41a4-0e5a-03a9ba585d00/public" width="500px"/>
11</div>
12
sqliteExplorerAppREADME.md1 match
3View and interact with your Val Town SQLite data. It's based off Steve's excellent [SQLite Admin](https://www.val.town/v/stevekrouse/sqlite_admin?v=46) val, adding the ability to run SQLite queries directly in the interface. This new version has a revised UI and that's heavily inspired by [LibSQL Studio](https://github.com/invisal/libsql-studio) by [invisal](https://github.com/invisal). This is now more an SPA, with tables, queries and results showing up on the same page.
45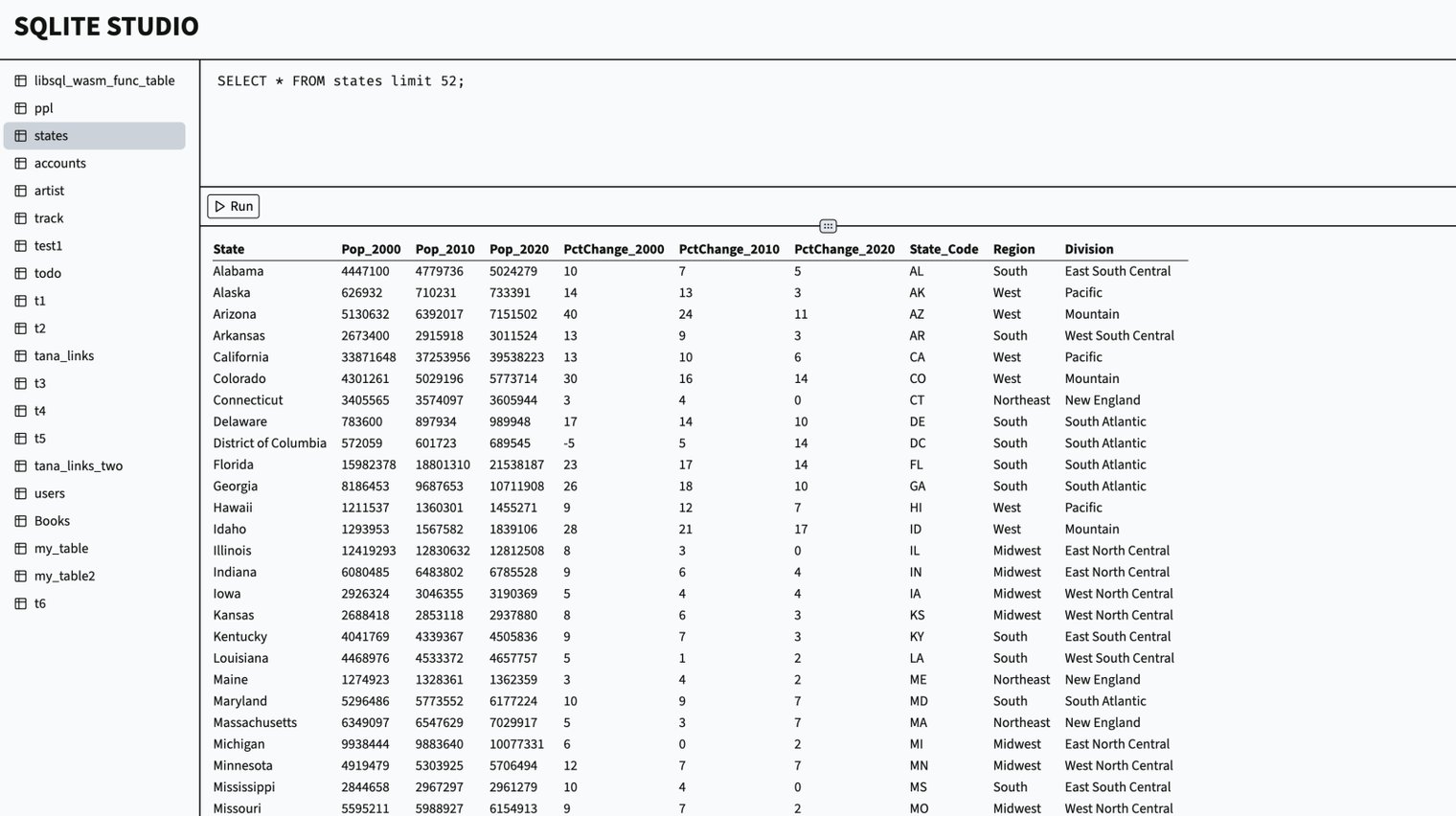
67## Install
valTownUsermain.tsx2 matches
35return userInfo?.bio;
36},
37async getProfileImageUrl() {
38const userInfo = await this.getUserInfo();
39return userInfo?.profileImageUrl;
40},
41async getEmail() {
geminiBboxREADME.md3 matches
1# Gemini AI Object Detection and Bounding Box Visualizer
23This application visualizes object detection results by drawing bounding boxes on images using the [Google's Gemini 1.5 Pro AI model](https://ai.google.dev/).
45Try using this image of 8 bananas, with 1 row and 1/2/4 columns: [https://f2.phage.directory/blogalog/bananas-8.png](https://f2.phage.directory/blogalog/bananas-8.png)
67Or this image of a phage plaque assay, with 3x3 grid and high contrast turned on: [https://f2.phage.directory/blogalog/pae7.png](https://f2.phage.directory/blogalog/pae7.png)
89This app is an adaptation of Simon Willison's idea, which you can read more about here: [https://simonwillison.net/2024/Aug/26/gemini-bounding-box-visualization/](https://simonwillison.net/2024/Aug/26/gemini-bounding-box-visualization/)
valTownDataLogsmain.tsx2 matches
8const email = await valTownUser.getEmail();
9const tier = await valTownUser.getTier();
10const profileImageUrl = await valTownUser.getProfileImageUrl();
1112console.log('User Information:');
16console.log(`Email: ${email}`);
17console.log(`Tier: ${tier}`);
18console.log(`Profile Image: ${profileImageUrl || 'Not set'}`);
1920console.log('\nEndpoint URLs:');