1/** @jsxImportSource https://esm.sh/react */
2import React, { useEffect, useRef, useState } from "https://esm.sh/react";
3import { createRoot } from "https://esm.sh/react-dom/client";
4
5function SoundMaker() {
1/** @jsxImportSource https://esm.sh/react */
2import React, { useState } from "https://esm.sh/react";
3import { createRoot } from "https://esm.sh/react-dom/client";
4
5function Card({ content }) {
1# Server-side Render React Mini Framework
2
3This is very experimental, more of a prototype of an architecture, than a true framework
1/** @jsxImportSource https://esm.sh/react */
2import { renderToString } from "https://esm.sh/react-dom@18.2.0/server";
3import { useEffect, useState } from "https://esm.sh/react@18.2.0";
4import { extractValInfo } from "https://esm.town/v/pomdtr/extractValInfo";
5import { html } from "https://esm.town/v/stevekrouse/html";
6
7// button that's disabled until client react hydrates
8export const Button = (props) => {
9 const [clientHydrated, setClientHydrated] = useState(false);
51 const props = await loader(req);
52 const script = `
53 import { hydrateRoot } from "https://esm.sh/react-dom@18.2.0/client";
54 import { jsx as _jsx } from "https://esm.sh/react@18.2.0/jsx-runtime";
55 import { Component } from "https://esm.town/v/${author}/${name}";
56
1# SSR React Mini & SQLite Todo App
2
3This Todo App is server rendered *and* client-hydrated React. This architecture is a lightweight alternative to NextJS, RemixJS, or other React metaframeworks with no compile or build step. The data is saved server-side in [Val Town SQLite](https://docs.val.town/std/sqlite/).
4
5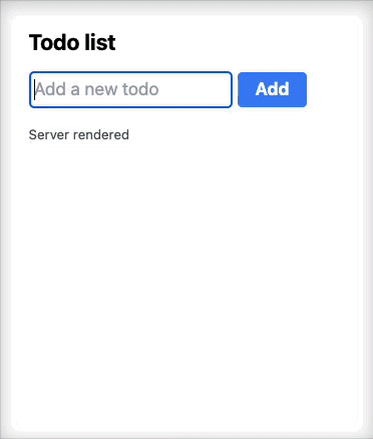
6
7## SSR React Mini Framework
8
9This ["framework"](https://www.val.town/v/stevekrouse/ssr_react_mini) is currently 44 lines of code, so it's obviously not a true replacement for NextJS or Remix.
10
11The trick is [client-side importing](https://www.val.town/v/stevekrouse/ssr_react_mini?v=53#L30) the React component that you're [server rendering](https://www.val.town/v/stevekrouse/ssr_react_mini?v=53#L35-37). Val Town is uniquely suited for this trick because it both runs your code server-side and exposes vals as [modules](https://esm.town/v/stevekrouse/TodoApp) importable by the browser.
12
13The tricky part is making sure that server-only code doesn't run on the client and vice-versa. For example, because this val colocates the server-side `loader` and `action` with the React component we have to be careful to do all server-only imports (ie sqlite) dynamically inside the [`loader`](https://www.val.town/v/stevekrouse/TodoApp?v=246#L7) and [`action`](https://www.val.town/v/stevekrouse/TodoApp?v=246#L20), so they only run server-side.
14
1/** @jsxImportSource https://esm.sh/react */
2import { zip } from "https://esm.sh/lodash-es";
3import { useEffect, useState } from "https://esm.sh/react@18.2.0";
4import codeOnValTown from "https://esm.town/v/andreterron/codeOnValTown?v=46";
5import { Form, hydrate } from "https://esm.town/v/stevekrouse/ssr_react_mini?v=75";
6
7export async function loader(req: Request) {
1# Daily Schedule & Chores Tracker
2A flexible and interactive analogue clock schedule and chore tracker designed for my 8-year-old. This React component helps manage daily routines and tasks, featuring a visually appealing clock interface and a dynamic chore list.
3
4## Features
11
12## Installation
13To use the component, include it in your React project and provide the necessary props.
14```ts
15import AnalogueScheduleClock from './path-to-component';
1/** @jsxImportSource https://esm.sh/react */
2import React, { useEffect, useMemo, useState } from "https://esm.sh/react";
3import { createRoot } from "https://esm.sh/react-dom/client";
4
5interface ScheduleItem {
63};
64
65const ScheduleSegment: React.FC<{
66 startMinutes: number;
67 endMinutes: number;
90};
91
92const ClockHand: React.FC<{ angle: number; length: number; width: number; color: string }> = (
93 { angle, length, width, color },
94) => (
115};
116
117const Legend: React.FC<{ schedule: ScheduleItem[]; currentTime: Date; heading: string }> = (
118 { schedule, currentTime, heading },
119) => {
150};
151
152const TodoList: React.FC<{ todos: TaskItem[]; heading: string }> = ({ todos, heading }) => {
153 const [todoItems, setTodoItems] = useState(todos);
154
1/** @jsxImportSource https://esm.sh/react */
2import { Cursors, init } from "https://esm.sh/@instantdb/react?bundle-deps";
3import React, { JSX } from "https://esm.sh/react";
4import { createRoot } from "https://esm.sh/react-dom/client";
5
6const cursorsClassNames =
1/** @jsxImportSource https://esm.sh/react */
2import { Cursors, init } from "https://esm.sh/@instantdb/react";
3import React from "https://esm.sh/react";
4import { createRoot } from "https://esm.sh/react-dom/client";
5
6function InstantCursors() {