1// JSX can be used in the client val thanks to this magic comment
2/** @jsxImportSource https://esm.sh/react **/
3// Make sure to only import from esm.sh (npm: specifier are not supported in the browser)
4import { motion } from "https://esm.sh/framer-motion";
5import React from "https://esm.sh/react";
6import ReactDOM from "https://esm.sh/react-dom";
7// import "https://esm.sh/tldraw/tldraw.css";
8
24
25// The app will be rendered inside the root div
26const root = ReactDOM.createRoot(document.getElementById("root"));
27root.render(<App />);
1/**
2 * @title Running React on the Client
3 * @description Vals can also be used to host client-side code!
4 * @preview https://pomdtr-react_example_server.web.val.run
5 * @include pomdtr/react_example_client
6 * @resource [React - Quick Start](https://react.dev/learn)
7 */
8
12 `<html>
13 <head>
14 <title>React Example</title>
15 <meta name="viewport" content="width=device-width, initial-scale=1" />
16 <style>
60 <body>
61 <main id="root"></main>
62 <script type="module" src="https://esm.town/v/tfayyaz/react_framer_motion_script"></script/>
63 </body>
64 </html>`,
1/**
2 * @title Running React on the Client
3 * @description Vals can also be used to host client-side code!
4 * @preview https://pomdtr-react_example_server.web.val.run
5 * @include pomdtr/react_example_client
6 * @resource [React - Quick Start](https://react.dev/learn)
7 */
8
12 `<html>
13 <head>
14 <title>React Example</title>
15 <meta name="viewport" content="width=device-width, initial-scale=1" />
16 <style>
21 <body>
22 <main id="root"></main>
23 <script type="module" src="https://esm.town/v/andreterron/react_tldraw_client"></script/>
24 </body>
25 </html>`,
1// JSX can be used in the client val thanks to this magic comment
2/** @jsxImportSource https://esm.sh/react **/
3// Make sure to only import from esm.sh (npm: specifier are not supported in the browser)
4import React from "https://esm.sh/react";
5import ReactDOM from "https://esm.sh/react-dom";
6import { Tldraw } from "https://esm.sh/tldraw";
7
18
19// The app will be rendered inside the root div
20const root = ReactDOM.createRoot(document.getElementById("root"));
21root.render(<App />);
1// JSX can be used in the client val thanks to this magic comment
2/** @jsxImportSource https://esm.sh/react **/
3// Make sure to only import from esm.sh (npm: specifier are not supported in the browser)
4import React from "https://esm.sh/react";
5import ReactDOM from "https://esm.sh/react-dom";
6import { Button } from "https://esm.town/v/nbbaier/shadcnButton";
7import {
53
54// The app will be rendered inside the root div
55const root = ReactDOM.createRoot(document.getElementById("root"));
56root.render(<App />);
1/** @jsxImportSource npm:preact */
2import { RenderFunc } from "https://esm.town/v/pomdtr/invoice_schema";
3import clsx from "npm:clsx";
4import { render as preactRender } from "npm:preact-render-to-string";
5
6export const render: RenderFunc = ({ title, table, to, from, details }) => {
7 return preactRender(
8 <html>
9 <head>
1# SSR React Mini & SQLite Todo App
2
3This Todo App is server rendered *and* client-hydrated React. This architecture is a lightweight alternative to NextJS, RemixJS, or other React metaframeworks with no compile or build step. The data is saved server-side in [Val Town SQLite](https://docs.val.town/std/sqlite/).
4
5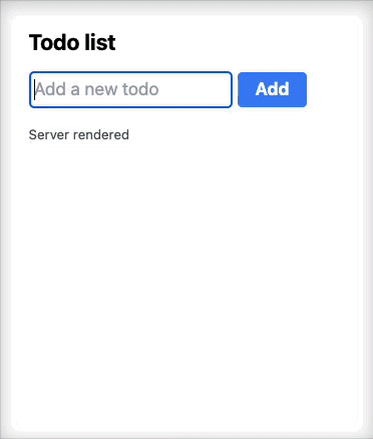
6
7## SSR React Mini Framework
8
9This ["framework"](https://www.val.town/v/stevekrouse/ssr_react_mini) is currently 44 lines of code, so it's obviously not a true replacement for NextJS or Remix.
10
11The trick is [client-side importing](https://www.val.town/v/stevekrouse/ssr_react_mini?v=53#L30) the React component that you're [server rendering](https://www.val.town/v/stevekrouse/ssr_react_mini?v=53#L35-37). Val Town is uniquely suited for this trick because it both runs your code server-side and exposes vals as [modules](https://esm.town/v/stevekrouse/TodoApp) importable by the browser.
12
13The tricky part is making sure that server-only code doesn't run on the client and vice-versa. For example, because this val colocates the server-side `loader` and `action` with the React component we have to be careful to do all server-only imports (ie sqlite) dynamically inside the [`loader`](https://www.val.town/v/stevekrouse/TodoApp?v=246#L7) and [`action`](https://www.val.town/v/stevekrouse/TodoApp?v=246#L20), so they only run server-side.
14
1/** @jsxImportSource https://esm.sh/react */
2import { zip } from "https://esm.sh/lodash-es";
3import { useEffect, useState } from "https://esm.sh/react@18.2.0";
4import codeOnValTown from "https://esm.town/v/andreterron/codeOnValTown?v=46";
5import { Form, hydrate } from "https://esm.town/v/stevekrouse/ssr_react_mini?v=75";
6
7export async function loader(req: Request) {
1# Simple HTML page
2
3with react-dom/server
4
1Basic demo of getting reactive Alpine.js working on a hono/jsx "backend"
2- For server <> frontend interaction, do form POST submissions (or potentially POST json to the public val address?)
3
4https://alpinejs.dev/start-here#building-a-counter
5
6Migrated from folder: Experiments/alpine/honoJsxAlpineReactive