1/** @jsxImportSource npm:react */
2import { api } from "https://esm.town/v/pomdtr/api";
3import { extractValInfo } from "https://esm.town/v/pomdtr/extractValInfo";
4import { html } from "https://esm.town/v/stevekrouse/html?v=5";
5import Markdoc from "npm:@markdoc/markdoc";
6import React from "npm:react";
7import { renderToString } from "npm:react-dom/server";
8
9const tags = {
25}
26
27export async function markdocReactExample(req: Request) {
28 // extract the current val readme
29 const { author, name } = extractValInfo(import.meta.url);
33 const ast = Markdoc.parse(readme);
34 const content = Markdoc.transform(ast, { tags }); // register the val tag
35 const rendered = Markdoc.renderers.react(content, React, {
36 components: {
37 Val,
1# [Markdoc Playground](https://pomdtr-markdocReactExample.web.val.run)
2
3This readme is rendered using [markdoc](https://markdoc.dev/).
4
5{% val author="pomdtr" name="markdocReactExample" %}
14## Frontend form
15
16You should have a form that hits the `/send-verification` API endpoint on submit. **Remember to adjust the endpoint URL to that of your fork** (or else you'll be signing people up for my website!). As a simple alternative, you could use the `/` handler of this Val, which returns a simple HTML form. Here's a simple example using React:
17
18```tsx
66## Frontend confirmation page
67
68Create a confirmation page that accepts an email and token as query params and calls the `/confirm-verification` endpoint. Simple example using React (and Next.js /page directory):
69
70```tsx
10You can override them if you wish to disallow CORS.
11
12This val is a client-side-rendered React app that makes requests to @stevekrouse/cors_example_backend. The backend is in a different val because CORS applies to requests on different domains. The backend has examples of the default permissive CORS behavior and disabled CORS.
13
14Migrated from folder: Archive/cors_example
1/** @jsxImportSource https://esm.sh/preact */
2import { render } from "npm:preact-render-to-string";
3
4export const remarkDemoJSX = async (req: Request) =>
1import { reactExample } from "https://esm.town/v/stevekrouse/reactExample?v=0";
2import { aFunction } from "https://esm.town/v/taufiq/aFunction";
3
4export async function aHandler(request: Request): Promise<Response> {
5 return reactExample();
6}
1# SSR React Mini & SQLite Todo App
2
3This Todo App is server rendered *and* client-hydrated React. This architecture is a lightweight alternative to NextJS, RemixJS, or other React metaframeworks with no compile or build step. The data is saved server-side in [Val Town SQLite](https://docs.val.town/std/sqlite/).
4
5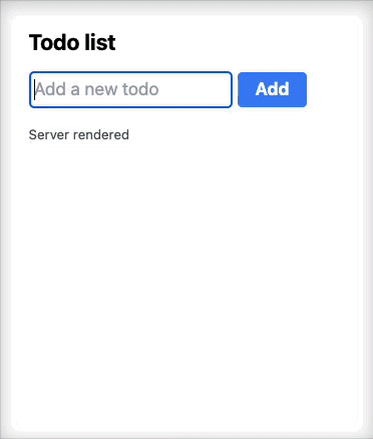
6
7## SSR React Mini Framework
8
9This ["framework"](https://www.val.town/v/stevekrouse/ssr_react_mini) is currently 44 lines of code, so it's obviously not a true replacement for NextJS or Remix.
10
11The trick is [client-side importing](https://www.val.town/v/stevekrouse/ssr_react_mini?v=53#L30) the React component that you're [server rendering](https://www.val.town/v/stevekrouse/ssr_react_mini?v=53#L35-37). Val Town is uniquely suited for this trick because it both runs your code server-side and exposes vals as [modules](https://esm.town/v/stevekrouse/TodoApp) importable by the browser.
12
13The tricky part is making sure that server-only code doesn't run on the client and vice-versa. For example, because this val colocates the server-side `loader` and `action` with the React component we have to be careful to do all server-only imports (ie sqlite) dynamically inside the [`loader`](https://www.val.town/v/stevekrouse/TodoApp?v=246#L7) and [`action`](https://www.val.town/v/stevekrouse/TodoApp?v=246#L20), so they only run server-side.
14
15
1# Example Full-stack Todo List App with React SSR + Client-side hydration & sqlite
2
3Requires you to put the React component in another val, in this case: https://www.val.town/v/stevekrouse/TodoApp
4
5Migrated from folder: Archive/TodoListApp/todo_list
1Migrated from folder: Archive/docsExamples/jsx/reactExample
1/** @jsxImportSource https://esm.sh/react */
2import { renderToString } from "npm:react-dom/server";
3
4export const reactExample = () =>
5 new Response(renderToString(<div>Test {1 + 1}</div>), {
6 headers: {
Starter template with client-side React & Hono server
A web-based dice roller using React on Val Town
Write business logic with ease
Meet the new standard for modern TypeScript development.
Type-safe, reactive, framework-agnostic library to manage your business logic.
Follow me if you learn more about JavaScript | TypeScript | React.js | Next.js | Linux | NixOS | Frontend Developer | https://linktr.ee/officialrajdeepsingh