mediumToMarkdownmain.tsx1 match
117118case 4:
119const url = `https://cdn-images-1.medium.com/max/${metadata.originalWidth * 2}/${metadata.id}`;
120121return ``;
falImageHandlermain.tsx1 match
1011const result: any = await fal.run("fal-ai/fast-lightning-sdxl", { input: { prompt } });
12return Response.json({ url: result.images[0].url });
13}
1import { generateImage } from "https://esm.town/v/isidentical/falImageGen?v=11";
2import { blob } from "https://esm.town/v/std/blob?v=12";
34const genKey = (key: string): string => {
5return "genImageCache-v1-" + key;
6};
710let url = await blob.getJSON(genKey(key));
11if (!url) {
12let resp = await generateImage(`generate the image you would expect if the url path was: "${key}"`);
13url = resp.url;
14await blob.setJSON(genKey(key), url);
15}
16return new Response((await fetch(url)).body, { headers: { "content-type": "image/jpg" } });
17}
falImageGenmain.tsx4 matches
1/**
2* Generates an image with Stable Diffusion XL through fal.ai
3*
4* @param {string} prompt - The input prompt to send to SDXL model.
5* @returns {Promise<string>} A promise that resolves to the URL of the image.
6*/
7export async function generateImage(
8input: string,
9): Promise<{ url: string }> {
10let resp = await fetch("https://isidentical-falimagehandler.web.val.run/", {
11method: "POST",
12body: JSON.stringify({ prompt: input }),
1# Image generated from a path name powered by fal.ai
23https://maxm-imggenurl.web.val.run/firefly.jpg
EditProfilePagemain.tsx14 matches
27title?: string;
28author?: string;
29coverImage?: string;
30};
31currentlyWatching?: {
32title?: string;
33platform?: string;
34posterImage?: string;
35};
36profile_img?: string;
72<div className="flex flex-col gap-4">
73<div>
74<label htmlFor="profile_img" className="text-sm font-medium text-neutral-800">Profile Image</label>
75<input
76id="profile_img"
77type="file"
78name="profile_img"
79accept="image/*"
80className="py-2"
81/>
201</div>
202<div>
203<label htmlFor="currentlyReadingCoverImage" className="text-sm font-medium text-neutral-800">Currently Reading Cover Image URL</label>
204<input
205id="currentlyReadingCoverImage"
206type="text"
207name="currentlyReadingCoverImage"
208value={user.currentlyReading?.coverImage}
209className="w-full mt-1 px-4 py-2 border rounded-md focus:outline-none focus:ring-2 focus:ring-blue-500"
210placeholder="Currently Reading Cover Image URL"
211/>
212</div>
234</div>
235<div>
236<label htmlFor="currentlyWatchingPosterImage" className="text-sm font-medium text-neutral-800">Currently Watching Poster Image URL</label>
237<input
238id="currentlyWatchingPosterImage"
239type="text"
240name="currentlyWatchingPosterImage"
241value={user.currentlyWatching?.posterImage}
242className="w-full mt-1 px-4 py-2 border rounded-md focus:outline-none focus:ring-2 focus:ring-blue-500"
243placeholder="Currently Watching Poster Image URL"
244/>
245</div>
6const userResult = await sqlite.execute({
7sql: `SELECT id, name, bio, username, email, location, currently_listening, currently_reading_title,
8currently_reading_author, currently_reading_cover_image, currently_watching_title,
9currently_watching_platform, currently_watching_poster_image, profile_theme, updated_at, profile_img
10FROM users WHERE username = ?`,
11args: [username],
16}
1718const [id, name, bio, usernameRetrieved, email, location, currentlyListening, currentlyReadingTitle, currentlyReadingAuthor, currentlyReadingCoverImage, currentlyWatchingTitle, currentlyWatchingPlatform, currentlyWatchingPosterImage, profile_theme, updated_at, profile_img] = userResult.rows[0];
1920const linksResult = await sqlite.execute({
25const links = linksResult.rows.map(([id, label, url]) => ({ id, label, url }));
2627return { id, name, bio, username: usernameRetrieved, email, location, currentlyListening, currentlyReading: { title: currentlyReadingTitle || '', author: currentlyReadingAuthor || '', coverImage: currentlyReadingCoverImage || '' }, currentlyWatching: { title: currentlyWatchingTitle || '', platform: currentlyWatchingPlatform || '', posterImage: currentlyWatchingPosterImage || '' }, links, profile_theme, updated_at, profile_img };
28}
2939await sqlite.execute({
40sql: `UPDATE users SET name = ?, bio = ?, location = ?, currently_listening = ?, currently_reading_title = ?,
41currently_reading_author = ?, currently_reading_cover_image = ?, currently_watching_title = ?,
42currently_watching_platform = ?, currently_watching_poster_image = ?, profile_theme = ?,
43updated_at = CURRENT_TIMESTAMP WHERE id = ? profile_img = ?`,
44args: [name, bio, location || null, currentlyListening || null, currentlyReading?.title || null, currentlyReading?.author || null, currentlyReading?.coverImage || null, currentlyWatching?.title || null, currentlyWatching?.platform || null, currentlyWatching?.posterImage || null, profile_theme || null, profile_img || userId],
45});
46}
121122123export const uploadProfileImage = async (file, username) => {
124const blobKey = `profile_img/${username}`;
125const reader = new FileReader();
140141142export async function getProfileImageUrl(username) {
143const key = `profile_img/${username}`;
144const imageUrl = await blob.get(key);
145return imageUrl;
146}
147
profileHandlersmain.tsx6 matches
1/** @jsxImportSource https://esm.sh/hono@latest/jsx **/
2import { getUserByUsername, updateUser, uploadProfileImage } from "https://esm.town/v/iamseeley/Queries";
3import EditProfilePage from "https://esm.town/v/iamseeley/EditProfilePage";
4import RootLayout from "https://esm.town/v/iamseeley/RootLayout";
41title: body.currentlyReadingTitle,
42author: body.currentlyReadingAuthor,
43coverImage: body.currentlyReadingCoverImage,
44};
45const currentlyWatching = {
46title: body.currentlyWatchingTitle,
47platform: body.currentlyWatchingPlatform,
48posterImage: body.currentlyWatchingPosterImage,
49};
50const profile_theme = body.profile_theme;
56const existingUser = await getUserByUsername(username);
5758// Handle profile image upload
59const profileImgFile = body.profile_img;
60let profileImgUrl = existingUser.profile_img; // Default to existing image URL
6162if (profileImgFile && profileImgFile.size) {
63profileImgUrl = await uploadProfileImage(profileImgFile, username);
64}
65
blob_adminREADME.md1 match
3This is a lightweight Blob Admin interface to view and debug your Blob data.
45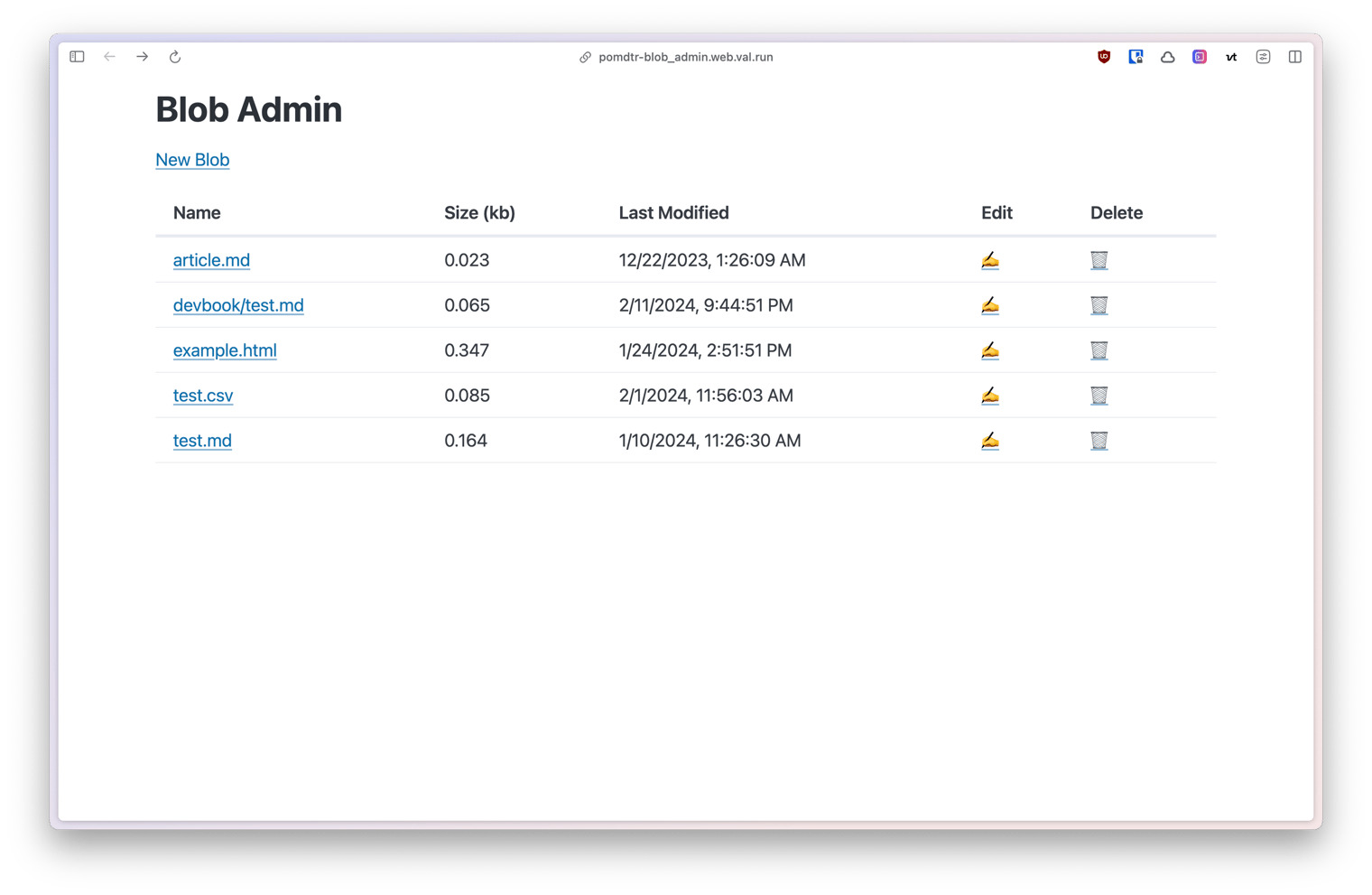
67Use this button to install the val:
reactExampleREADME.md1 match
1Fancy animated SVGs in readmes, along with centering and image sizing.
2```
3<div align="center"><img width=200 src="https://gpanders.com/img/DEC_VT100_terminal.jpg"></div>